#include<iostream> using namespace std; class Shape { public: virtual double area() const=0; }; class Circle:public Shape { public: Circle(double r):radius(r) { } virtual double area() const { return 3.14159*radius*radius; } protected: double radius; }; class Square:public Shape { public: Square(double s):side(s) { } virtual double area() const { return side*side; } protected: double side; }; class Rectangle:public Shape { public: Rectangle(double w,double h):width(w),height(h) { } virtual double area() const { return width*height; } protected: double width,height; }; class Trapezoid:public Shape { public: Trapezoid(double t,double b,double h):top(t),bottom(b),height(h) { } virtual double area() const { return 0.5*(top+bottom)*height; } protected: double top,bottom,height; }; class Triangle:public Shape { public: Triangle(double w,double h):width(w),height(h) { } virtual double area() const { return 0.5*width*height; } protected: double width,height; }; int main() { Circle circle(12.6); Square square(3.5); Rectangle rectangle(4.5,8.4); Trapezoid trapezoid(2.0,4.5,3.2); Triangle triangle(4.5,8.4); Shape *pt[5]= { &circle,&square,&rectangle,&trapezoid,&triangle }; double areas=0.0; for(int i=0; i<5; i++) { areas=areas+pt[i]->area(); } cout<<"total of all areas="<<areas<<endl; return 0; }这段代码若要输出Circle:498.51 Square:12.25 Rectangle:37.80 Trapezoid:10.40 Triangle:18.90,如何改进
时间: 2023-12-15 10:16:13 浏览: 90
可以将输出语句放在循环中,分别输出每个图形的面积。可以在每个 Shape 类的子类中添加一个成员函数,用于返回该图形的名称,然后在输出语句中使用该函数获取图形名称。代码如下:
```
#include<iostream>
using namespace std;
class Shape {
public:
virtual double area() const = 0;
virtual string name() const = 0; // 添加获取图形名称的函数
};
class Circle : public Shape {
public:
Circle(double r) : radius(r) {}
virtual double area() const {
return 3.14159 * radius * radius;
}
virtual string name() const { // 返回图形名称
return "Circle";
}
protected:
double radius;
};
class Square : public Shape {
public:
Square(double s) : side(s) {}
virtual double area() const {
return side * side;
}
virtual string name() const {
return "Square";
}
protected:
double side;
};
class Rectangle : public Shape {
public:
Rectangle(double w, double h) : width(w), height(h) {}
virtual double area() const {
return width * height;
}
virtual string name() const {
return "Rectangle";
}
protected:
double width, height;
};
class Trapezoid : public Shape {
public:
Trapezoid(double t, double b, double h) : top(t), bottom(b), height(h) {}
virtual double area() const {
return 0.5 * (top + bottom) * height;
}
virtual string name() const {
return "Trapezoid";
}
protected:
double top, bottom, height;
};
class Triangle : public Shape {
public:
Triangle(double w, double h) : width(w), height(h) {}
virtual double area() const {
return 0.5 * width * height;
}
virtual string name() const {
return "Triangle";
}
protected:
double width, height;
};
int main() {
Circle circle(12.6);
Square square(3.5);
Rectangle rectangle(4.5, 8.4);
Trapezoid trapezoid(2.0, 4.5, 3.2);
Triangle triangle(4.5, 8.4);
Shape* pt[] = { &circle,&square,&rectangle,&trapezoid,&triangle };
double areas = 0.0;
for (int i = 0; i < 5; i++) {
cout << pt[i]->name() << ":" << pt[i]->area() << " "; // 输出图形名称和面积
areas += pt[i]->area();
}
cout << endl << "total of all areas=" << areas << endl;
return 0;
}
```
阅读全文
相关推荐
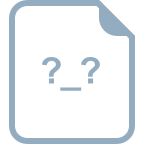
















