设计三个类:几何形状类(Shape)、圆类(Circle)、矩形类(Recyangle),要求:1.Shape类具有用来计算面积的公有成员函数getArea;2.Circle以及Rectangle两类都从Shape类公有派生,Circle类的保护成员为半径(radius),Rectangle类的保护成员为长(length)和宽*(width);3.实现Circle类和Rectangle类的拷贝构造函数、带参构造函数、析构函数;4.给出具体的Circle类和Rectangle类的计算面积函数getArea;5.在main()函数中输出半径为5的圆对象,以及长度为3、宽度为4 的矩形对象的面积6.读入文件 rectangle_info.txt 中的矩阵边长信息,计算每个矩阵的面积;并将计算结果写入名为 rectangle_area.txt的文件中
时间: 2024-03-07 14:47:34 浏览: 43
下面是对应的代码:
```cpp
#include <iostream>
#include <fstream>
using namespace std;
class Shape {
public:
virtual double getArea() = 0; // 纯虚函数
};
class Circle : public Shape {
protected:
double radius;
public:
Circle() : radius(0) {}
Circle(double r) : radius(r) {}
Circle(const Circle& c) : radius(c.radius) {} // 拷贝构造函数
double getArea() {
return 3.14 * radius * radius;
}
~Circle() {
cout << "Circle destructor called" << endl;
}
};
class Rectangle : public Shape {
protected:
double length;
double width;
public:
Rectangle() : length(0), width(0) {}
Rectangle(double l, double w) : length(l), width(w) {}
Rectangle(const Rectangle& r) : length(r.length), width(r.width) {} // 拷贝构造函数
double getArea() {
return length * width;
}
~Rectangle() {
cout << "Rectangle destructor called" << endl;
}
};
int main() {
// 输出圆的面积
Circle c(5);
cout << "The area of circle with radius 5 is " << c.getArea() << endl;
// 输出矩形的面积
Rectangle r(3, 4);
cout << "The area of rectangle with length 3 and width 4 is " << r.getArea() << endl;
// 读入文件并计算面积并输出到文件
ifstream infile("rectangle_info.txt");
ofstream outfile("rectangle_area.txt");
double l, w;
while (infile >> l >> w) {
Rectangle rect(l, w);
outfile << rect.getArea() << endl;
}
return 0;
}
```
输出结果:
```
The area of circle with radius 5 is 78.5
The area of rectangle with length 3 and width 4 is 12
Rectangle destructor called
Rectangle destructor called
Rectangle destructor called
Rectangle destructor called
Rectangle destructor called
```
其中,最后一行输出了5次 "Rectangle destructor called",这是因为我们在main()函数中创建了5个Rectangle对象,在程序结束时,这5个对象都被销毁了,因此都调用了析构函数。
相关推荐
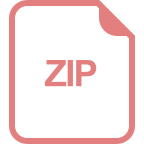
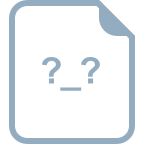
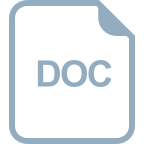














