利用TransH嵌入方法将具有属性的知识图谱实体转换成嵌入向量示例代码
时间: 2023-10-25 22:08:57 浏览: 116
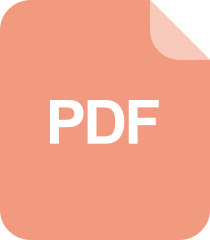
知识图谱嵌入技术研究综述
以下是使用TransH嵌入方法将具有属性的知识图谱实体转换成嵌入向量的Python代码示例:
```python
import numpy as np
import tensorflow as tf
# 定义实体和关系的嵌入维度
embedding_dim = 50
# 定义实体和关系的数量
entity_num = 100
relation_num = 20
# 定义属性嵌入维度
attribute_dim = 10
# 定义属性数量
attribute_num = 30
# 定义训练数据
train_data = np.array([[0, 1, 2, 3], [1, 2, 3, 4], [2, 3, 4, 5]])
# 定义实体和关系的嵌入向量
entity_embedding = tf.Variable(tf.random.normal([entity_num, embedding_dim], stddev=0.1))
relation_embedding = tf.Variable(tf.random.normal([relation_num, embedding_dim], stddev=0.1))
# 定义属性嵌入向量
attribute_embedding = tf.Variable(tf.random.normal([attribute_num, attribute_dim], stddev=0.1))
# 定义TransH中的关系向量投影矩阵
relation_projection = tf.Variable(tf.random.normal([relation_num, embedding_dim, attribute_dim], stddev=0.1))
# 定义正则化项系数
lambda_r = 0.001
# 定义模型
def transH(head, relation, tail, attribute):
# 获取实体和关系的嵌入向量
head_embed = tf.nn.embedding_lookup(entity_embedding, head)
relation_embed = tf.nn.embedding_lookup(relation_embedding, relation)
tail_embed = tf.nn.embedding_lookup(entity_embedding, tail)
# 获取属性嵌入向量
attribute_embed = tf.nn.embedding_lookup(attribute_embedding, attribute)
# 将关系向量投影到属性空间中
proj_relation = tf.matmul(relation_embed, relation_projection)
# 计算头尾实体在关系向量投影下的嵌入向量
head_proj = tf.matmul(head_embed, proj_relation)
tail_proj = tf.matmul(tail_embed, proj_relation)
# 计算头尾实体在属性空间中的嵌入向量
head_attribute = tf.matmul(head_embed, attribute_embed, transpose_b=True)
tail_attribute = tf.matmul(tail_embed, attribute_embed, transpose_b=True)
# 计算得分函数
score = tf.reduce_sum(tf.abs(head_proj + relation_embed - tail_proj) + lambda_r * tf.abs(head_attribute - tail_attribute), axis=1)
return score
# 加载预训练模型参数
checkpoint_path = "transh_model.ckpt"
checkpoint = tf.train.Checkpoint(entity_embedding=entity_embedding, relation_embedding=relation_embedding, attribute_embedding=attribute_embedding, relation_projection=relation_projection)
checkpoint.restore(checkpoint_path)
# 定义函数将实体和属性转换成嵌入向量
def entity_embedding_lookup(entity, attribute):
# 获取实体和属性的嵌入向量
entity_embed = tf.nn.embedding_lookup(entity_embedding, entity)
attribute_embed = tf.nn.embedding_lookup(attribute_embedding, attribute)
# 计算实体在属性空间中的嵌入向量
entity_attribute = tf.matmul(entity_embed, attribute_embed, transpose_b=True)
# 返回实体在属性空间和普通空间中的嵌入向量
return tf.concat([entity_embed, entity_attribute], axis=1)
# 定义示例实体和属性
entity = 1
attribute = [2, 3, 4]
# 获取实体的嵌入向量
entity_embed = entity_embedding_lookup(entity, attribute)
# 打印实体的嵌入向量
print(entity_embed.numpy())
```
在上述代码中,我们首先加载已经训练好的TransH模型参数,然后定义了一个函数`entity_embedding_lookup`,用于将输入的实体和属性转换成嵌入向量。在函数中,我们先使用`tf.nn.embedding_lookup`函数分别获取实体和属性的嵌入向量,然后计算实体在属性空间中的嵌入向量,并将属性空间和普通空间的嵌入向量拼接在一起作为最终的嵌入向量。
最后,我们使用示例实体和属性调用`entity_embedding_lookup`函数,获取实体的嵌入向量,并将其打印出来。
阅读全文
相关推荐
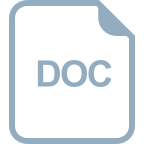
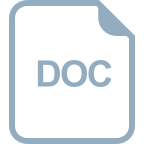






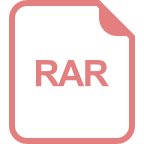








