使用python读取选中的png格式图片,将图中的表格转化为可编辑的excel表格,并将表格保存在桌面,运用opencv库和pandas库
时间: 2023-05-21 18:01:02 浏览: 171
可以使用以下代码实现:
```python
import cv2
import pandas as pd
# 读取图片
img = cv2.imread('selected_image.png')
# 将图片转化为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 对灰度图进行二值化处理
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 查找表格轮廓
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 获取表格轮廓的矩形框坐标
table_contours = []
for cnt in contours:
x, y, w, h = cv2.boundingRect(cnt)
if w > 100 and h > 100:
table_contours.append((x, y, w, h))
# 将表格转化为pandas的DataFrame
dfs = []
for i, (x, y, w, h) in enumerate(table_contours):
table_img = img[y:y+h, x:x+w]
table_gray = cv2.cvtColor(table_img, cv2.COLOR_BGR2GRAY)
table_thresh = cv2.threshold(table_gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
table_data = pd.read_csv(pd.compat.StringIO(pd.read_table(pd.compat.StringIO(table_thresh.tostring().decode('utf-8')), header=None).to_csv(header=None, index=None)), header=None)
dfs.append(table_data)
# 将多个DataFrame合并为一个
result = pd.concat(dfs)
# 将结果保存为excel文件
result.to_excel('Desktop/result.xlsx', index=None, header=None)
```
这段代码使用了OpenCV库和Pandas库,可以将选中的PNG格式图片中的表格转化为可编辑的Excel表格,并将表格保存在桌面。
相关推荐
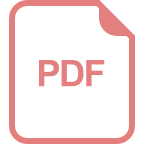
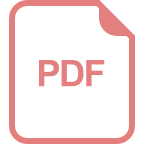
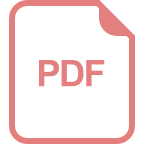














