对三分类数据进行smote的Python代码
时间: 2024-01-02 12:04:09 浏览: 85
以下是对三分类数据进行SMOTE的Python代码:
```python
from imblearn.over_sampling import SMOTE
from sklearn.datasets import make_classification
# 生成三分类数据
X, y = make_classification(n_classes=3, class_sep=2,
weights=[0.1, 0.3, 0.6], n_informative=3,
n_redundant=1, flip_y=0, n_features=20,
n_clusters_per_class=1, n_samples=1000,
random_state=10)
# 使用SMOTE对数据进行过采样
smote = SMOTE(random_state=42)
X_res, y_res = smote.fit_resample(X, y)
```
在这个例子中,我们使用了`make_classification`函数生成了一个具有3个类别的数据集。然后,我们使用了`SMOTE`函数对数据进行了过采样,并将结果保存在`X_res`和`y_res`中。
相关问题
smote算法python代码
以下是使用smote算法进行过抽样的样例代码:
首先,需要安装imbalanced-learn库,可以通过以下命令安装:
```
!pip install imbalanced-learn
```
然后,可以使用以下代码实现smote算法的过抽样:
```python
from imblearn.over_sampling import SMOTE
from sklearn.datasets import make_classification
X, y = make_classification(n_classes=2, class_sep=2,
weights=[0.1, 0.9], n_informative=3,
n_redundant=1, flip_y=0, n_features=20,
n_clusters_per_class=1, n_samples=1000,
random_state=10)
print('Original dataset shape %s' % Counter(y))
# 使用SMOTE算法进行过抽样
sm = SMOTE(random_state=42)
X_res, y_res = sm.fit_resample(X, y)
print('Resampled dataset shape %s' % Counter(y_res))
```
这里使用了imbalanced-learn库中的SMOTE类进行过抽样。需要注意的是,这里使用的是make_classification函数生成的数据集,实际应用中需要使用自己的数据集。
4分类,smote过采样python代码
SMOTE(Synthetic Minority Over-sampling Technique)是一种常用的过采样技术,用于处理不平衡数据集,特别适用于少数类别样本较多的情况。在Python中,你可以使用`imblearn`库中的`SMOTE`类来进行这个操作。下面是一个简单的例子:
```python
from imblearn.over_sampling import SMOTE
import pandas as pd
from sklearn.datasets import load_iris
# 加载示例数据集,这里假设我们有一个DataFrame df
# df = ...
# 提取特征和目标变量
X = df.drop('target_column', axis=1) # 将'target_column'替换为你的真实目标列名
y = df['target_column']
# 初始化SMOTE对象
smote = SMOTE()
# 应用SMOTE对X进行过采样
X_resampled, y_resampled = smote.fit_resample(X, y)
# 现在你可以将X_resampled和y_resampled分别用于训练模型
```
在这个代码中,`fit_resample()`方法会返回一个新的、过采样的特征集和标签集。记得先安装`imblearn`库,如果还没有安装,可以使用`pip install imblearn`命令。
阅读全文
相关推荐


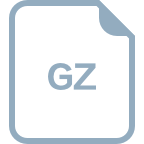













