nodejs操作mongodb
时间: 2023-10-08 11:10:09 浏览: 45
Node.js提供了许多不同的方式来操作MongoDB数据库。以下是其中一些常见的方法:
1.使用官方的MongoDB Node.js驱动程序
可以使用官方的MongoDB Node.js驱动程序来连接和操作MongoDB数据库。这个驱动程序提供了许多不同的方法和选项,可以满足不同的需求。以下是一个简单的例子,展示如何使用官方驱动程序连接到MongoDB数据库,并执行简单的查询操作:
```
const MongoClient = require('mongodb').MongoClient;
// Connection URL
const url = 'mongodb://localhost:27017';
// Database Name
const dbName = 'myproject';
// Use connect method to connect to the server
MongoClient.connect(url, function(err, client) {
console.log("Connected successfully to server");
const db = client.db(dbName);
// Find some documents
db.collection('users').find({}).toArray(function(err, docs) {
console.log("Found the following records");
console.log(docs);
client.close();
});
});
```
2.使用Mongoose库
Mongoose是一个MongoDB对象模型工具,它提供了一种更简单的方式来操作MongoDB数据库。它允许您定义模式和模型,以便更轻松地执行常见的操作,如插入、更新和查询文档。以下是一个例子,展示如何使用Mongoose连接到MongoDB数据库,并定义一个简单的用户模型:
```
const mongoose = require('mongoose');
// Connection URL
const url = 'mongodb://localhost:27017/myproject';
// Connect to the database
mongoose.connect(url, {useNewUrlParser: true});
// Define a schema
const userSchema = new mongoose.Schema({
name: String,
email: String,
age: Number
});
// Define a model
const User = mongoose.model('User', userSchema);
// Create a new user
const user = new User({
name: 'John Smith',
email: 'john@example.com',
age: 30
});
// Save the user to the database
user.save(function(err) {
if (err) throw err;
// Find all users
User.find(function(err, users) {
if (err) throw err;
console.log(users);
mongoose.connection.close();
});
});
```
3.使用第三方库
除了官方驱动程序和Mongoose之外,还有许多其他的Node.js库可以用于操作MongoDB数据库。一些流行的库包括MongoDB Native、mongojs和Monk。这些库提供了不同的API和功能,可以满足不同的需求。以下是一个例子,展示如何使用mongojs库连接到MongoDB数据库,并执行简单的查询操作:
```
const mongojs = require('mongojs');
// Connection URL
const url = 'mongodb://localhost:27017/myproject';
// Connect to the database
const db = mongojs(url, ['users']);
// Find all users
db.users.find(function(err, users) {
if (err) throw err;
console.log(users);
db.close();
});
```
无论您选择使用哪种方法,Node.js提供了许多选项和库,可以帮助您轻松地操作MongoDB数据库。
相关推荐
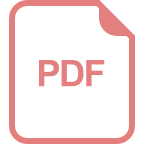














