c# 二维装箱问题 箱子大小固定
时间: 2023-11-12 15:10:16 浏览: 280
二维装箱问题是一个经典的计算机科学问题,它涉及到如何将一些矩形物体装进一个固定大小的矩形容器中,使得所有物体都能够被容器包含,同时尽可能地节省容器的空间。
在C#中,可以使用贪心算法来解决二维装箱问题。具体来说,可以按照矩形物体的面积从大到小的顺序对它们进行排序,然后依次将每个物体放置在容器中。当一个物体无法放入当前容器时,就创建一个新的容器,并将该物体放入其中。
以下是一个简单的C#程序,用于实现二维装箱问题的贪心算法:
```csharp
using System;
using System.Collections.Generic;
class Rectangle
{
public int Width { get; set; }
public int Height { get; set; }
public Rectangle(int width, int height)
{
Width = width;
Height = height;
}
public int GetArea()
{
return Width * Height;
}
}
class Container
{
public int Width { get; set; }
public int Height { get; set; }
public Container(int width, int height)
{
Width = width;
Height = height;
}
public bool CanFit(Rectangle rectangle)
{
return rectangle.Width <= Width && rectangle.Height <= Height;
}
}
class Program
{
static void Main(string[] args)
{
List<Rectangle> rectangles = new List<Rectangle>()
{
new Rectangle(3, 4),
new Rectangle(5, 2),
new Rectangle(2, 3),
new Rectangle(4, 4),
new Rectangle(1, 5),
new Rectangle(6, 1)
};
int containerCount = 1;
List<Container> containers = new List<Container>() { new Container(8, 8) };
rectangles.Sort((r1, r2) => r2.GetArea() - r1.GetArea());
foreach (Rectangle rectangle in rectangles)
{
bool isPlaced = false;
foreach (Container container in containers)
{
if (container.CanFit(rectangle))
{
isPlaced = true;
container.Width -= rectangle.Width;
container.Height -= rectangle.Height;
break;
}
}
if (!isPlaced)
{
containerCount++;
containers.Add(new Container(8, 8));
containers[containerCount - 1].Width -= rectangle.Width;
containers[containerCount - 1].Height -= rectangle.Height;
}
}
Console.WriteLine($"需要 {containerCount} 个容器");
}
}
```
在上述程序中,我们首先定义了一个`Rectangle`类来表示矩形物体,以及一个`Container`类来表示容器。然后,我们创建了一个包含一些矩形物体的列表,并按照面积从大到小的顺序对它们进行了排序。接下来,我们创建了一个空的容器列表,并将一个初始容器添加到其中。然后,我们遍历每个矩形物体,并尝试将其放入当前容器中。如果当前容器无法容纳该物体,则创建一个新的容器,并将该物体放入其中。最后,我们输出需要的容器数量。
请注意,在上述程序中,我们假设容器的大小为8x8,但你可以根据自己的需求修改容器的大小。同时,该算法并不能保证得到最优解,但通常可以得到较好的近似解。
阅读全文
相关推荐
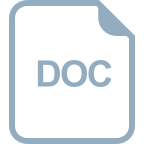
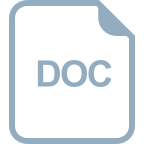
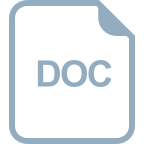



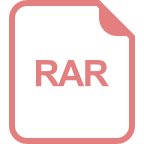
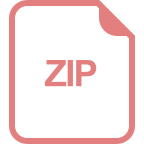
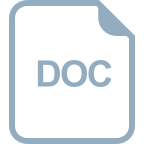
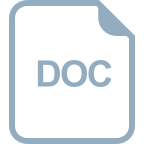
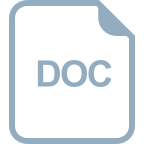
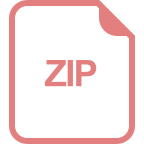
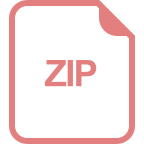
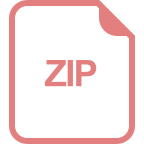
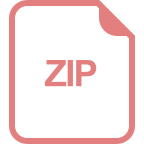
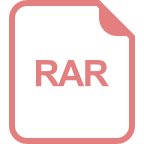
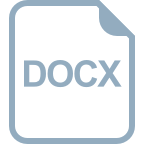
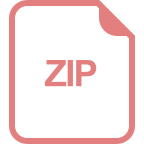