实现一个简易的购物车 需求:利用vue实现简易的购物车功能,能够进行商品数量的加减,商品种类的删除,商品价格的计算。用<template>,一共5条信息,并且用方框隔起来
时间: 2024-10-14 22:02:52 浏览: 23
为了实现一个简单的Vue购物车功能,我们可以创建一个基本的组件结构,包含商品列表、操作按钮(增加、减少、删除)以及总价计算。下面是一个简化的`<template>`部分的示例:
```html
<template>
<div class="shopping-cart">
<div v-for="(item, index) in cartItems" :key="index" style="display: flex; border: 1px solid #ccc; padding: 10px; margin-bottom: 10px;">
<span>商品名称: {{ item.name }}</span>
<span>数量: <input type="number" :value="item.quantity" @input="updateQuantity(index)" /></span>
<button @click="decrementQuantity(index)">-</button>
<button @click="incrementQuantity(index)">+</button>
<button @click="removeItem(index)">删除</button>
<!-- 商品价格计算 -->
<p>价格: {{ calculatePrice(item.price) }}</p>
</div>
<h3>总计: {{ totalPrice }}</h3>
</div>
</template>
```
在这个模板中:
- `cartItems` 是一个Vue数据属性,用于存储购物车的商品列表。
- `<v-for>` 循环遍历每个商品,显示商品名称、数量输入框和操作按钮。
- `@input` 和 `@click` 事件监听器分别用于数量修改和增减操作,需要在相应的`methods`里定义`updateQuantity`, `decrementQuantity`, 和 `incrementQuantity`函数。
- `removeItem` 函数用于从列表中移除商品。
- `calculatePrice` 是一个方法,接收单价并返回总价。
接下来,你需要在`script`部分添加相关的Vue实例和数据,以及上述提到的方法:
```javascript
<script>
export default {
data() {
return {
cartItems: [
{ name: '商品A', quantity: 1, price: 10 },
{ name: '商品B', quantity: 2, price: 20 },
// ... 其他商品
],
totalPrice: 0,
};
},
methods: {
updateQuantity(index, event) {
const newQuantity = parseInt(event.target.value);
this.cartItems[index].quantity = newQuantity;
this.calculateTotal();
},
decrementQuantity(index) {
this.cartItems[index].quantity--;
if (this.cartItems[index].quantity <= 0) {
this.removeItem(index);
} else {
this.calculateTotal();
}
},
incrementQuantity(index) {
this.cartItems[index].quantity++;
this.calculateTotal();
},
removeItem(index) {
this.cartItems.splice(index, 1);
this.calculateTotal();
},
calculatePrice(price) {
return price * this.cartItems.find(item => item.name === '当前商品').quantity;
},
calculateTotal() {
this.totalPrice = this.cartItems.reduce((total, item) => total + item.price * item.quantity, 0);
},
},
};
</script>
```
阅读全文
相关推荐
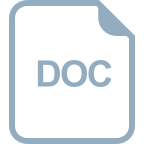
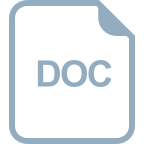
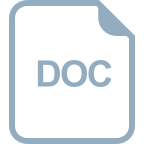

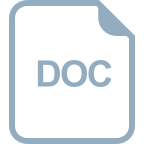
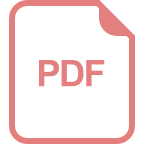
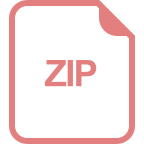
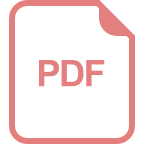
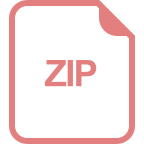
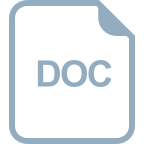
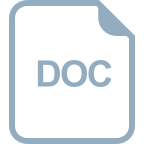
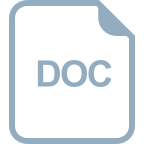
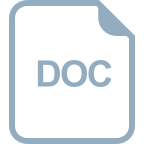




