python apply applymap agg
时间: 2023-10-14 08:03:11 浏览: 46
Python中的apply、applymap和agg都是用于对DataFrame数据进行操作的函数。
首先,apply函数是用于对DataFrame的行或列进行一元操作的方法。它可以接受一个函数作为参数,并对每一行或每一列调用该函数,返回一个结果。例如,可以使用apply函数计算每一列的均值、求和等。apply函数的应用比较灵活,可以自己定义函数或使用lambda表达式。需要注意的是,apply函数默认是按列进行操作,如果想按行进行操作,需要指定axis参数为1。
其次,applymap函数是用于对DataFrame中的每一个元素进行操作的方法。它可以接受一个函数作为参数,并对每一个元素进行调用,返回一个结果。例如,可以使用applymap函数将所有元素转换为大写字母。与apply函数不同的是,applymap函数只能用于DataFrame,不能用于Series。
最后,agg函数是用于对DataFrame的多列进行聚合操作的方法。它可以接受一个函数或函数列表作为参数,并对指定的多个列进行操作,返回一个结果。例如,可以使用agg函数计算多个列的均值、求和等。agg函数相比于apply函数,更适合对多列进行聚合操作。
综上所述,apply、applymap和agg都是非常实用的DataFrame操作函数,可以根据需求对行、列或多列进行元素级别的操作和聚合操作。
相关问题
python apply和applymap函数
Python中的apply和applymap函数都是用于对数据进行操作的函数。
apply函数可以用于对DataFrame中的某一列或某一行进行操作,可以传入一个函数作为参数,该函数会被应用到每一行或每一列上,返回一个Series对象。
applymap函数可以用于对DataFrame中的每一个元素进行操作,可以传入一个函数作为参数,该函数会被应用到每一个元素上,返回一个新的DataFrame对象。
两个函数的区别在于apply函数是对一列或一行进行操作,而applymap函数是对每一个元素进行操作。
apply applymap
"apply" 和 "applymap" 是 Pandas 库中的两方法,用于对数据进行操作和转换。
1. "apply" 方法用于对 DataFrame 的一行或一列进行操作。它接受一个函数作为参数,并将该函数应用于每个元素或行/列。可以将自定义函数或 Lambda 函数传递给 "apply" 方法。
例如,假设有一个 DataFrame df,我们想将每个元素取平方根,可以使用以下代码:
```
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
def square_root(x):
return x ** 0.5
df = df.apply(square_root)
```
这将返回一个新的 DataFrame,其中每个元素都被取了平方根。
2. "applymap" 方法用于对 DataFrame 的每个元素应用相同的函数。与 "apply" 方法不同,它不能用于行或列。同样,可以传递自定义函数或 Lambda 函数给 "applymap" 方法。
例如,我们想将 DataFrame 的所有元素转换为字符串格式,可以使用以下代码:
```
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df = df.applymap(str)
```
这将返回一个新的 DataFrame,其中每个元素都被转换为字符串格式。
总结起来,"apply" 方法用于对 DataFrame 的行或列进行操作,而 "applymap" 方法用于对 DataFrame 的每个元素进行操作。
相关推荐
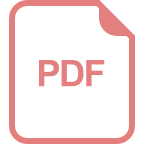
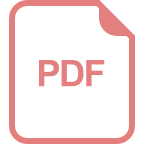












