c++实现图书管理系统代码
时间: 2024-08-14 13:00:19 浏览: 39
在C++中实现一个简单的图书管理系统,我们可以创建一个基础的设计,包括书籍类(Book),图书馆类(Library)以及用户类(User)。这里是一个基本的框架示例:
```cpp
#include <iostream>
#include <vector>
// 书籍类
class Book {
public:
std::string title;
std::string author;
int quantity;
// 构造函数
Book(const std::string& title, const std::string& author, int quantity)
: title(title), author(author), quantity(quantity) {}
// 显示书的信息
void displayInfo() {
std::cout << "Title: " << title << ", Author: " << author << ", Quantity: " << quantity << std::endl;
}
};
// 图书馆类
class Library {
private:
std::vector<Book> books;
public:
void addBook(Book book) {
books.push_back(book);
}
void removeBook(const std::string& title) {
for (auto it = books.begin(); it != books.end(); ++it) {
if (it->title == title) {
books.erase(it);
break;
}
}
}
// 其他方法如搜索书籍等...
};
// 用户类
class User {
public:
void borrowBook(const std::string& title) {
for (const auto& book : library.books) {
if (book.title == title && book.quantity > 0) {
book.quantity--;
std::cout << "Borrowed: " << book.title << std::endl;
break;
}
}
}
void returnBook(const std::string& title) {
for (auto& book : library.books) {
if (book.title == title) {
book.quantity++;
std::cout << "Returned: " << book.title << std::endl;
break;
}
}
}
private:
Library library;
};
int main() {
Book book1("The Great Gatsby", "F. Scott Fitzgerald", 5);
Book book2("To Kill a Mockingbird", "Harper Lee", 3);
Library lib;
lib.addBook(book1);
lib.addBook(book2);
User user;
user.library = lib;
// 用户操作
user.borrowBook("The Great Gatsby");
user.returnBook("To Kill a Mockingbird");
return 0;
}
```
相关推荐
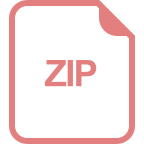
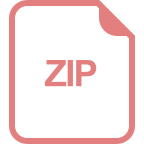
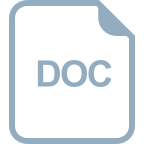
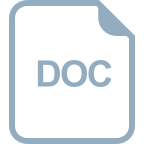
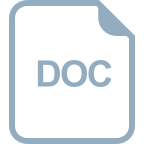
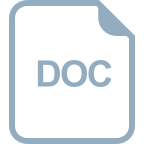
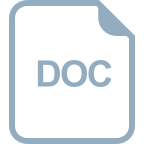
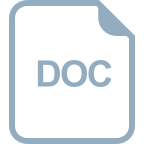
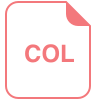
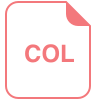







