对脑电信号采用STFT提取特征分类的python实例
时间: 2024-09-10 17:09:55 浏览: 23
短时傅里叶变换(STFT)是一种分析非平稳信号的常用方法,它通过将信号分解为一系列短时间窗口上的频谱,从而得到信号随时间变化的频率信息。在脑电信号(EEG)处理中,STFT可以用于提取时间-频率特征,这些特征随后可以用于分类等机器学习任务。
以下是一个简单的Python实例,展示了如何使用STFT来提取脑电信号特征,并为后续的分类任务准备数据:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.signal import stft, spectrogram
from sklearn.preprocessing import StandardScaler
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
from mne import create_info, EpochsArray
# 假设我们有一个二维数组data,其中每一行代表一个脑电通道的时间序列数据
# data.shape == (通道数, 时间点数)
data = np.random.randn(32, 1000) # 生成一个随机的脑电信号数据
# 创建一个模拟的事件数组,用于定义分类标签(例如,0或1)
labels = np.array([0, 1]) # 假设有两种不同的状态或类别
# 将脑电信号数据转换为mne的EpochsArray对象,方便后续处理
info = create_info(ch_names=range(data.shape[0]), sfreq=100, ch_types='eeg')
epochs = EpochsArray(data, info, tmin=0, verbose=False)
# 定义STFT参数
n_fft = 512 # 傅里叶变换的点数
fs = 100 # 采样频率
# 对每个通道进行STFT
stft_features = []
for ch_idx in range(data.shape[0]):
# 对当前通道数据进行STFT
f, t, Zxx = stft(data[ch_idx, :], fs=fs, n_fft=n_fft, return_onesided=True)
# 从STFT结果中提取特征,例如取模后的幅值
feature_matrix = np.abs(Zxx) # 转换为幅度谱
# 将特征矩阵展平,以便于后续处理
stft_features.append(feature_matrix.flatten())
# 将特征堆叠起来,形成一个二维数组,每行对应一个样本的特征向量
stft_features = np.vstack(stft_features)
# 将标签与特征对齐
label_idx = 0
X = []
y = []
for label in labels:
X.append(stft_features[label_idx])
y.append(label)
label_idx += len(data) // len(labels)
X = np.array(X)
y = np.array(y)
# 数据标准化
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X_scaled, y, test_size=0.2, random_state=42)
# 使用随机森林分类器进行训练
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)
# 对测试集进行分类预测
y_pred = clf.predict(X_test)
# 打印分类准确率
print('分类准确率:', np.mean(y_pred == y_test))
```
在这个实例中,我们首先模拟生成了一个脑电信号数据集,然后使用STFT来提取每个通道的时间-频率特征,并将特征展平后用于训练随机森林分类器。最后,我们在测试集上评估了分类器的性能。
相关推荐
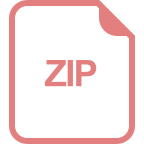
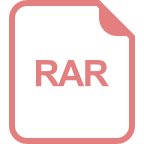
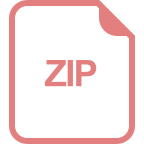














