unity game 游戏预览怎么移动相机
时间: 2023-12-28 09:02:15 浏览: 30
在Unity游戏中,要移动相机以预览游戏场景,可以通过编写脚本来实现。首先,需要在Unity编辑器中创建一个新的C#脚本,然后将其附加到主相机对象上。在脚本中,可以使用Input.GetAxis函数来捕捉玩家输入的移动方向。例如,可以使用该函数获取玩家的鼠标或手柄输入,并将其转换成相机的移动方向。
通过改变相机的transform.position属性可以实现相机的移动。可以在脚本中通过修改相机的x、y、z坐标来调整其位置。如果希望相机视角朝向游戏场景的特定区域,还可以使用transform.LookAt函数来实现。这个函数可以让相机自动调整朝向游戏场景中任何指定的目标。
另外,还可以在脚本中添加一些控制相机移动的限制条件,例如防止相机移出游戏场景边界或避免相机移动到地图以外的区域。这些限制可以通过一些条件语句和数学运算来实现,以确保相机始终在合适的位置移动。
最后,需要在Unity编辑器中启用脚本,然后即可在游戏中通过玩家输入来移动相机进行游戏场景的预览。这样,就能为玩家提供更加灵活的游戏预览体验。
相关问题
unity 运行情况下移动相机脚本
### 回答1:
在Unity中,要在运行情况下移动相机,我们可以编写一个相机移动的脚本。
首先,我们需要在场景中创建一个相机对象,并将此脚本添加到相机对象上。接下来,在脚本中声明一些私有变量,用于控制相机的移动。
我们可以使用Input.GetAxis函数来获取用户输入的移动方向。例如,我们可以使用"Horizontal"和"Vertical"来获取水平和垂直方向上的输入。
在Update函数中,我们可以使用上述输入值来计算相机的目标位置。例如,我们可以使用transform.Translate函数来移动相机的位置。我们可以将相机的位置设置为当前位置与移动方向的乘积,以实现相机的移动。
为了使相机平滑移动,我们可以使用Lerp函数来插值相机的位置。这需要使用一个目标位置和一个插值系数,在每帧更新时,将当前位置移向目标位置。
在脚本中,还可以添加一些逻辑,例如限制相机的移动范围,或者添加其他控制逻辑。
最后,我们需要将此脚本附加到相机对象上,并在Unity中启动游戏进行测试。在游戏运行情况下,我们可以通过输入控制相机的移动,并观察相机的实时位置变化。
通过编写和调试相机移动脚本,我们可以实现在Unity中运行情况下移动相机的功能。
### 回答2:
Unity运行时移动相机有多种方法,可以使用脚本来实现。以下是一个简单的相机移动脚本示例,可以通过键盘输入来控制相机的移动。
首先,在Unity中创建一个新的C#脚本,然后将其命名为"CameraMovement"。
在脚本中,我们需要声明一些变量,用于控制相机的移动速度和灵敏度。
```c#
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraMovement : MonoBehaviour
{
public float movementSpeed = 5f;
public float rotationSpeed = 5f;
// Update is called once per frame
void Update()
{
// 相机的前后移动
float translation = Input.GetAxis("Vertical") * movementSpeed;
translation *= Time.deltaTime;
transform.Translate(0, 0, translation);
// 相机的左右旋转
float rotation = Input.GetAxis("Horizontal") * rotationSpeed;
rotation *= Time.deltaTime;
transform.Rotate(0, rotation, 0);
}
}
```
在脚本中的Update函数中,我们根据用户输入的按键来移动相机。通过使用Input.GetAxis函数获取水平方向和垂直方向的输入值,然后乘以移动速度和灵敏度,再乘以Time.deltaTime,以确保移动的平滑性。
上面的脚本将通过键盘的上下箭头键控制相机的前进后退,并使用键盘的左右箭头键控制相机的左右旋转。
要使该脚本生效,将其附加到Unity场景中的相机对象上。然后,当您在Unity编辑器中点击“播放”按钮时,您就可以使用键盘来移动和旋转相机了。
这只是一个基本的相机移动脚本示例,您可以根据需要进行修改和扩展,以实现更复杂的相机移动效果。
### 回答3:
Unity是一款强大的游戏引擎,可以轻松创建各种类型的游戏。在Unity中,我们可以通过编写脚本来控制相机的移动。
在Unity中移动相机有很多种方法,其中一种常见的方法是使用脚本来控制相机的位置和旋转。下面是一个简单的示例脚本:
```c#
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraMovement : MonoBehaviour
{
public float speed = 5f; // 相机移动速度
void Update()
{
// 获取键盘输入
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
// 根据输入移动相机的位置
Vector3 movement = new Vector3(moveHorizontal, 0f, moveVertical) * speed * Time.deltaTime;
transform.position += movement;
// 控制相机旋转
if (Input.GetKey(KeyCode.Q))
{
transform.Rotate(Vector3.up, -speed * Time.deltaTime);
}
else if (Input.GetKey(KeyCode.E))
{
transform.Rotate(Vector3.up, speed * Time.deltaTime);
}
}
}
```
上述代码中,我们首先定义了一个`speed`变量来控制相机的移动速度。在`Update()`函数中,我们使用`Input.GetAxis()`函数来获取键盘的输入值,然后根据输入移动相机的位置,其中`Time.deltaTime`是一个时间因子,可以保证在不同帧率下相机移动的速度相同。
此外,我们还可以使用`Input.GetKey()`函数来检测按键,并使用`Transform.Rotate()`函数来控制相机的旋转。
通过编写类似的脚本,我们就可以在Unity的运行环境中移动相机,实现自定义相机的控制功能。
unity 根据鼠标移动相机
在Unity中,我们可以使用鼠标移动来控制相机的移动。下面是一种实现思路:
首先,我们需要在Unity中创建一个脚本来实现相机的移动。我们可以把脚本附加到相机上,或者新建一个空物体作为摄像机的父物体,然后把脚本附加到父物体上。
在脚本中,我们需要定义一些变量来控制相机的移动速度,以及鼠标移动的灵敏度。我们可以使用Input.GetAxis函数来获取鼠标在水平和垂直方向上的移动距离。
然后,我们可以在Update函数中使用这些变量来控制相机的移动。我们可以通过修改相机的transform.position属性来改变相机的位置。具体来说,我们可以使用Vector3的加法运算来计算新的相机位置,其中我们将鼠标移动距离乘以移动速度和灵敏度作为偏移量。
最后,我们需要对相机的移动范围进行限制,以防止相机移动到不允许的区域。我们可以使用Mathf.Clamp函数来限制相机的位置在指定的范围内。这样可以确保相机只在规定的区域内移动。
通过这种方法,我们可以实现鼠标移动相机的功能。这样,当我们在运行Unity场景时,通过鼠标的移动,可以改变相机的位置,从而达到控制相机的效果。
相关推荐
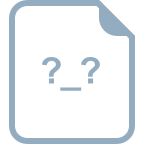












