如何用C语言编程计算阶乘之和的倒数?
时间: 2024-11-13 14:26:37 浏览: 10
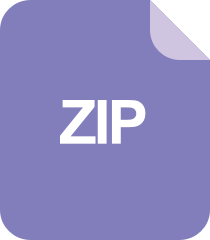
c代码-10.功能:用while语句计算1到n的倒数之和。
在C语言中,我们可以编写一个程序来计算阶乘之和的倒数。首先,我们需要一个函数来计算阶乘,然后计算阶乘数组的所有元素之和,最后计算这个和的倒数。下面是一个简单的步骤说明:
1. 定义一个函数`factorial_sum(n)`来计算从1到n的所有整数的阶乘之和。这通常会使用循环,并在每次迭代中更新总和。
```c
unsigned long long factorial_sum(int n) {
unsigned long long sum = 0;
for (int i = 1; i <= n; ++i) {
sum += factorial(i);
}
return sum;
}
unsigned long long factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
```
2. 计算阶乘之和后,计算其倒数。由于可能得到非常大的数值,可能会溢出浮点数,因此可以使用long double类型并考虑近似值。
```c
long double inv_factorial_sum(unsigned long long sum) {
const long double epsilon = 1e-9; // 设置一个较小的误差容忍度
long double reciprocal = 1 / sum;
if (fabs(reciprocal) < epsilon) {
printf("Warning: Division by zero or very small number.\n");
return 0; // 或者返回适当的近似值
} else {
return reciprocal;
}
}
```
3. 最后,在主程序中调用上述函数并输出结果。
```c
int main() {
int n;
printf("Enter a positive integer: ");
scanf("%d", &n);
unsigned long long total_sum = factorial_sum(n);
long double inverse = inv_factorial_sum(total_sum);
printf("The reciprocal of the sum of factorials from 1 to %d is approximately %.5Lf.\n", n, inverse);
return 0;
}
```
阅读全文
相关推荐
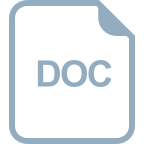
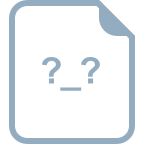
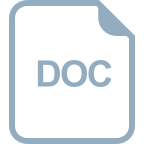
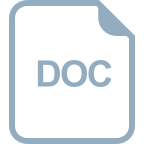




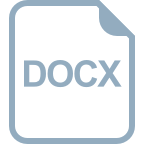
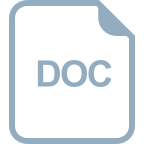
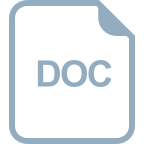
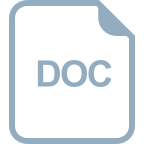
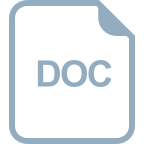
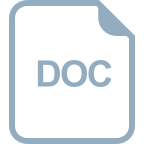
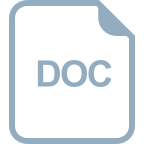
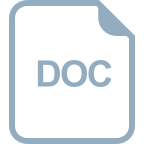

