凯撒密码如何用C语言实现
时间: 2024-09-28 13:11:04 浏览: 26
凯撒密码是一种简单的替代密码技术,它通过将明文中的每个字母按照字母表向右或向左移动固定数量的位置来加密。在C语言中,我们可以编写一个简单的函数来实现这个过程。这里是一个基本的例子,我们只考虑小写字母的加密:
```c
#include <stdio.h>
#include <ctype.h>
// 定义凯撒密码的移动步长
#define SHIFT 3
// 加密函数,输入是字符,输出是加密后的字符
char caesar_cipher(char c) {
if ('a' <= c && c <= 'z') { // 如果是小写字母
c = ((c - 'a' + SHIFT) % 26) + 'a'; // 向右移动步长
}
return c;
}
// 实际加密字符串的函数
void caesar_encrypt(const char *input, char *output) {
for (int i = 0; input[i]; ++i) {
output[i] = caesar_cipher(input[i]);
}
output[i] = '\0'; // 结束字符串并添加终止符
}
int main() {
char plaintext[50];
char ciphertext[50];
printf("Enter the plaintext: ");
fgets(plaintext, sizeof(plaintext), stdin); // 输入明文
plaintext[strcspn(plaintext, "\n")] = '\0'; // 去除换行符
caesar_encrypt(plaintext, ciphertext);
printf("Encrypted message: %s\n", ciphertext);
return 0;
}
```
在这个例子中,用户可以在`main()`函数中输入明文,程序会将其加密并打印出加密后的结果。
相关推荐
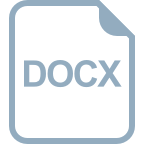
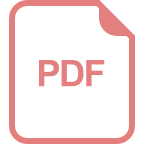
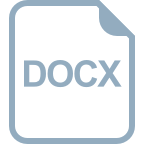














