用c语言编写,凯撒密码
时间: 2024-10-04 07:02:05 浏览: 10
凯撒密码(Caesar Cipher)是一种古老的加密技术,通过将字母表中的每个字符按照固定的位移进行替换来达到加密的目的。下面是一个简单的C语言版本的凯撒密码实现,我们将位移设置为3作为示例:
```c
#include <stdio.h>
#include <ctype.h>
// 函数:将明文转换成密文
void caesar_encrypt(char* plaintext, char* ciphertext, int shift) {
while (*plaintext != '\0') {
// 检查是否为大写字母、小写字母还是其他字符
if (isalpha(*plaintext)) {
// 对大写字母和小写字母做不同的偏移操作
if (isupper(*plaintext)) {
ciphertext[shift] = *plaintext + shift - 'A';
ciphertext += shift;
*plaintext = toupper(*plaintext);
} else {
ciphertext[shift] = *plaintext + shift - 'a';
ciphertext += shift;
*plaintext = tolower(*plaintext);
}
} else {
// 非字母字符保持不变
ciphertext[shift] = *plaintext;
ciphertext++;
plaintext++;
}
}
ciphertext[shift] = '\0'; // 结束字符串
}
int main() {
char plaintext[] = "Hello, World!";
char ciphertext[26];
// 设置加密位移
int shift = 3;
// 加密
caesar_encrypt(plaintext, ciphertext, shift);
printf("原文: %s\n", plaintext);
printf("密文: %s\n", ciphertext);
return 0;
}
```
在这个例子中,我们遍历输入的明文,如果是字母就将其向右移动`shift`位置,非字母字符保持不变。注意这个程序假设位移不大于25,因为英文大写字母最多只有26个。
相关推荐
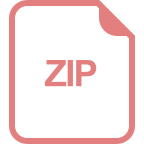
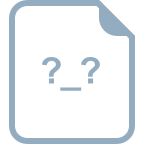
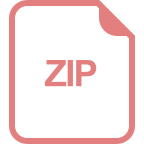

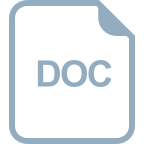


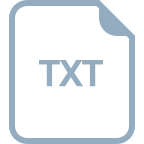
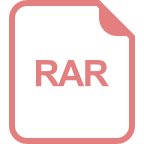
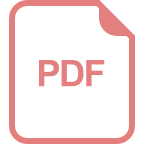
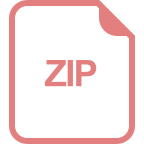
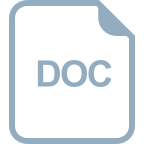




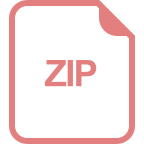
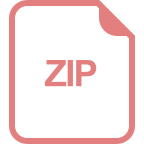