SD AttributeError: 'dict' object has no attribute 'shape'
时间: 2024-07-12 14:01:19 浏览: 341
在Python中,`AttributeError: 'dict' object has no attribute 'shape'` 这种错误通常是由于尝试在一个字典(dict)对象上执行一个只适用于数组(ndarray)或具有特定形状数据结构的方法,比如`shape`。`shape`属性是在NumPy库中的多维数组对象中用来获取数组维度信息的。
例如,如果你试图运行类似这样的代码:
```python
my_dict = {'a': [1, 2, 3], 'b': [4, 5]}
print(my_dict.shape)
```
你会看到这个错误,因为`my_dict`是一个普通的字典,并不是一个具有`shape`属性的数组。
解决这个问题的方法是确保你在正确的对象类型上调用相应的方法。如果需要检查元素的维度,你应该遍历字典并为每个值单独检查,而不是期望整个字典有一个形状:
```python
if isinstance(my_dict, dict):
for value in my_dict.values():
if hasattr(value, 'shape'):
print(value.shape)
```
相关问题
attributeerror: 'dict' object has no attribute 'shape'
根据提供的引用内容,'dict' object has no attribute 'shape'这个错误是因为字典类型没有'shape'属性。'shape'属性通常用于numpy数组中,用于获取数组的形状。因此,如果您在使用字典时遇到此错误,请检查您的代码并确保您正在使用正确的数据类型。
以下是一个例子,展示了如何使用numpy数组的'shape'属性来获取数组的形状:
```python
import numpy as np
# 创建一个3x3的数组
arr = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# 获取数组的形状
print(arr.shape) # 输出:(3, 3)
```
AttributeError: 'dict' object has no attribute 'shape'
AttributeError: 'dict' object has no attribute 'shape' 是一个常见的错误,它表示在一个字典对象上尝试访问 'shape' 属性时发生了错误。字典是 Python 中的一种数据结构,它由键值对组成。然而,字典对象并没有 'shape' 这个属性,因此当你尝试访问它时会引发 AttributeError。
通常情况下,'shape' 属性是用于表示数组或矩阵的维度信息的,例如在 NumPy 或 TensorFlow 中。如果你想获取一个数组或矩阵的形状信息,你需要使用相应的库函数或方法来获取。
以下是一些常见的获取数组或矩阵形状的方法:
1. 在 NumPy 中,你可以使用 `numpy.shape()` 函数来获取数组的形状。例如:
```
import numpy as np
arr = np.array([[1, 2, 3], [4, 5, 6]])
shape = np.shape(arr)
print(shape) # 输出 (2, 3)
```
2. 在 TensorFlow 中,你可以使用 `tensorflow.shape()` 方法来获取张量的形状。例如:
```
import tensorflow as tf
tensor = tf.constant([[1, 2, 3], [4, 5, 6]])
shape = tf.shape(tensor)
print(shape) # 输出 tf.Tensor([2 3], shape=(2,), dtype=int32)
```
请注意,在使用这些方法之前,你需要确保已经正确导入了相应的库。
阅读全文
相关推荐
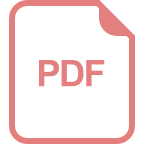












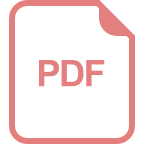

