实现C++的智能指针shared_ptr
时间: 2023-10-17 16:25:53 浏览: 46
要在 C 语言中实现类似 C++ 的智能指针 shared_ptr 的功能,你可以使用以下步骤:
1. 创建一个结构体来表示智能指针。该结构体应包含指向数据的指针以及一个引用计数器。
```c
typedef struct {
void* data;
int* refCount;
} shared_ptr;
```
2. 定义一个函数来创建共享指针并初始化引用计数器。
```c
shared_ptr* create_shared_ptr(void* data) {
shared_ptr* ptr = malloc(sizeof(shared_ptr));
ptr->data = data;
ptr->refCount = malloc(sizeof(int));
*(ptr->refCount) = 1;
return ptr;
}
```
3. 定义一个函数来增加引用计数。
```c
void increment_ref_count(shared_ptr* ptr) {
(*(ptr->refCount))++;
}
```
4. 定义一个函数来减少引用计数,并在引用计数为零时释放内存。
```c
void decrement_ref_count(shared_ptr* ptr) {
(*(ptr->refCount))--;
if (*(ptr->refCount) == 0) {
free(ptr->data);
free(ptr->refCount);
free(ptr);
}
}
```
5. 最后,可以使用这些函数来创建和管理共享指针对象。
```c
int main() {
int* data = malloc(sizeof(int));
*data = 42;
shared_ptr* ptr1 = create_shared_ptr(data);
increment_ref_count(ptr1);
shared_ptr* ptr2 = ptr1;
increment_ref_count(ptr2);
decrement_ref_count(ptr1);
decrement_ref_count(ptr2);
return 0;
}
```
这是一个简单的示例,演示了如何实现 C 语言中的共享指针。你可以根据自己的需要进行扩展和修改。
相关推荐
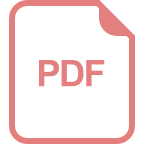
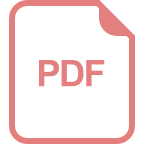
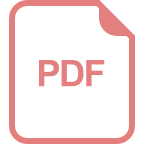














