FutureWarning: adjacency_matrix will return a scipy.sparse array instead of a matrix in Networkx 3.0. tem1 = nx.adjacency_matrix(G1)
时间: 2024-09-12 22:14:07 浏览: 152
`FutureWarning` 是Python中用于警告即将在未来的某个版本中更改或弃用某个功能的消息。在这个特定的情况下,`FutureWarning` 提示的是在Networkx库的下一个主要版本(3.0)中,`adjacency_matrix` 函数将不再返回一个`matrix`类型的对象,而是返回一个`scipy.sparse`的稀疏矩阵类型。这种变化主要是为了提高性能和内存使用效率,因为`scipy.sparse`矩阵通常比传统的二维数组更加高效。
在你的代码片段中:
```python
tem1 = nx.adjacency_matrix(G1)
```
这行代码调用`networkx`库中的`adjacency_matrix`函数来生成图`G1`的邻接矩阵。如果你使用的是Networkx的旧版本,那么返回的可能是一个`matrix`类型的对象。但是,当Networkx更新到3.0版本后,这个函数将会返回一个`scipy.sparse`类型的对象。
为了确保代码在未来版本的Networkx中也能正常工作,你可能需要修改代码,以兼容`scipy.sparse`矩阵的使用。这可能涉及到如何创建、访问和操作稀疏矩阵的知识。如果你不熟悉`scipy.sparse`,可能需要查看相关文档和教程,以便理解和应用这些改变。
相关问题
AttributeError: module 'networkx' has no attribute 'to_numpy_matrix'
This error occurs when you try to use the `to_numpy_matrix` function from the `networkx` module, but the function is not found. This may be due to using an older version of the module where the function was not yet implemented, or it may have been removed in a newer version of the module.
To fix this error, you can try updating the `networkx` module to the latest version, or you can use an alternative method to convert the graph to a numpy matrix. One option is to use the `adjacency_matrix` function from the `scipy` module, which can be used to create a sparse matrix representation of the graph. Another option is to manually create a numpy matrix by iterating over the nodes and edges of the graph.
计算下面复杂网络拓扑属性的代码:1邻接矩阵 2-每个节点的节点度 3.网络的平均节点度 4节点度分布 5-拉普拉斯矩阵 6网络密度 7-所有最短路径 8.每个节点的偏心度 9网络半径 10网络直径 11平均最短路径 12.每个节点的接近程度 13-找到并绘制混合模式的程度相关性 14-同时找到邻接矩阵和拉普拉斯矩阵的特征值和特征向量
这是一个十分复杂的问题,需要用到多个库和算法来实现。下面是一些可能用到的库和算法:
1. 邻接矩阵和节点度:使用 NetworkX 库来创建图形对象,然后使用其函数来计算邻接矩阵和节点度。
``` python
import networkx as nx
# 创建图形对象
G = nx.Graph()
# 添加节点和边
G.add_nodes_from([1, 2, 3])
G.add_edges_from([(1, 2), (2, 3)])
# 计算邻接矩阵和节点度
adj_matrix = nx.adjacency_matrix(G).todense()
degrees = dict(G.degree())
```
2. 平均节点度:使用 NetworkX 库中的函数来计算平均节点度。
``` python
avg_degree = sum(degrees.values()) / len(degrees)
```
3. 节点度分布:使用 Matplotlib 库来绘制节点度的分布图。
``` python
import matplotlib.pyplot as plt
# 获取节点度列表
degree_list = list(degrees.values())
# 绘制直方图
plt.hist(degree_list, bins=range(max(degree_list)+2))
plt.show()
```
4. 拉普拉斯矩阵:使用 Scipy 库中的函数来计算拉普拉斯矩阵。
``` python
import scipy.sparse as sp
# 计算拉普拉斯矩阵
laplacian = sp.csgraph.laplacian(adj_matrix)
```
5. 网络密度:使用 NetworkX 库中的函数来计算网络密度。
``` python
density = nx.density(G)
```
6. 所有最短路径:使用 NetworkX 库中的函数来计算所有最短路径。
``` python
shortest_paths = dict(nx.all_pairs_shortest_path(G))
```
7. 偏心度:使用 NetworkX 库中的函数来计算每个节点的偏心度。
``` python
eccentricities = nx.eccentricity(G)
```
8. 网络半径和直径:使用 NetworkX 库中的函数来计算网络的半径和直径。
``` python
radius = nx.radius(G)
diameter = nx.diameter(G)
```
9. 平均最短路径:使用 NetworkX 库中的函数来计算平均最短路径长度。
``` python
avg_shortest_path = nx.average_shortest_path_length(G)
```
10. 接近程度:使用 NetworkX 库中的函数来计算每个节点的接近程度。
``` python
closeness = nx.closeness_centrality(G)
```
11. 混合模式的程度相关性:使用 Pandas 库来加载数据集,并使用 Seaborn 库来绘制混合模式的程度相关性图。
``` python
import pandas as pd
import seaborn as sns
# 加载数据集
data = pd.read_csv('data.csv')
# 绘制混合模式的程度相关性图
sns.jointplot(x='degree', y='clustering', data=data, kind='hex')
```
12. 特征值和特征向量:使用 Scipy 库中的函数来计算邻接矩阵和拉普拉斯矩阵的特征值和特征向量。
``` python
import scipy.linalg as la
# 计算邻接矩阵和拉普拉斯矩阵的特征值和特征向量
adj_eigvals, adj_eigvecs = la.eig(adj_matrix)
lap_eigvals, lap_eigvecs = la.eig(laplacian)
```
阅读全文
相关推荐
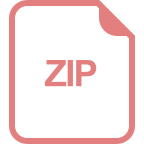
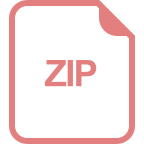
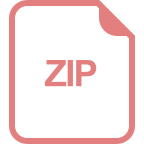
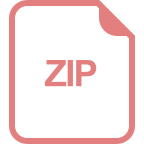
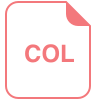
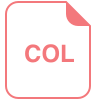
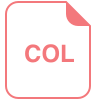
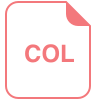
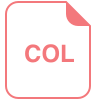
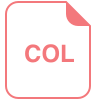
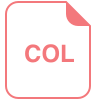
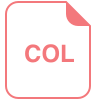
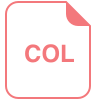
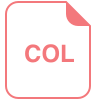
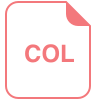
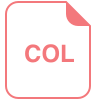
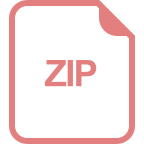