根据提示,在右侧编辑器Begin-End处补充代码,编写一个程序, 对用户录入的产品信息进行格式化。
时间: 2024-06-15 13:06:24 浏览: 93
Sure! 以下是一个示例程序,用于对用户录入的产品信息进行格式化:
```python
def format_product_info(product_name, product_price, product_quantity):
formatted_info = f"产品名称:{product_name}\n价格:¥{product_price}\n数量:{product_quantity}"
return formatted_info
# 用户输入产品信息
product_name = input("请输入产品名称:")
product_price = float(input("请输入产品价格:"))
product_quantity = int(input("请输入产品数量:"))
# 调用函数进行格式化
formatted_info = format_product_info(product_name, product_price, product_quantity)
# 打印格式化后的产品信息
print(formatted_info)
```
请注意,上述代码是使用Python编写的示例程序。用户需要依次输入产品名称、价格和数量,然后程序会将这些信息格式化并打印出来。
相关问题
在右侧编辑器begin-end处补充代码,根据输入数据绘制热成像图并隐藏坐标
要根据输入数据绘制热成像图并隐藏坐标,可以使用Python中的matplotlib库来实现。
首先,需要导入所需的库和模块:
import numpy as np
import matplotlib.pyplot as plt
然后,根据输入数据绘制热成像图,可以采用imshow函数:
data = np.random.rand(10, 10) # 假设输入数据为一个10x10的二维数组
plt.imshow(data, cmap='hot')
接着,隐藏坐标可以通过设置坐标轴的可见性来实现:
plt.gca().xaxis.set_visible(False)
plt.gca().yaxis.set_visible(False)
最后,在编辑器的begin-end处加入以上代码即可得到绘制热成像图并隐藏坐标的效果。完整代码如下:
import numpy as np
import matplotlib.pyplot as plt
data = np.random.rand(10, 10) # 假设输入数据为一个10x10的二维数组
plt.imshow(data, cmap='hot')
plt.gca().xaxis.set_visible(False)
plt.gca().yaxis.set_visible(False)
plt.show()
这样就可以根据输入数据绘制热成像图并隐藏坐标。
根据提示,在右侧编辑器 begin-end 区间补充代码,完成双向链表的初始化操作,插入操
双向链表是一种常用的数据结构,每个节点都有一个指向前一个节点和后一个节点的指针。下面是双向链表的初始化操作和插入操作的代码实现。
初始化操作:
```
// 定义双向链表节点的结构
class Node {
public:
int data; // 数据
Node* prev; // 指向前一个节点的指针
Node* next; // 指向后一个节点的指针
};
// 初始化双向链表
Node* initDoublyLinkedList() {
// 创建头节点
Node* head = new Node();
head->prev = nullptr;
head->next = nullptr;
// 返回头节点指针
return head;
}
```
插入操作:
```
// 在双向链表的指定位置插入新节点
void insertNode(Node* head, int position, int value) {
// 创建新节点
Node* newNode = new Node();
newNode->data = value;
// 寻找插入位置的节点
Node* currNode = head;
for (int i = 0; i < position; i++) {
if (currNode->next == nullptr) {
break; // 达到链表尾部
}
currNode = currNode->next;
}
// 修改指针,完成插入操作
if (currNode->next != nullptr) {
currNode->next->prev = newNode;
}
newNode->next = currNode->next;
currNode->next = newNode;
newNode->prev = currNode;
}
```
以上就是双向链表的初始化操作和插入操作的代码实现。初始化操作会创建一个空的双向链表,并返回头节点的指针。插入操作可以在指定位置插入新节点,并保持链表的完整性。
相关推荐
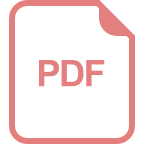
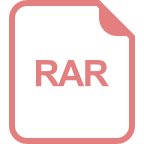
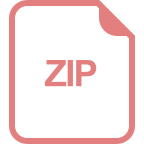
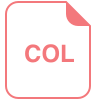











