逆波兰表达式用c++实现
时间: 2024-11-28 09:14:36 浏览: 56
逆波兰表达式(Reverse Polish Notation,RPN),也称为后缀表达式,是一种将操作数放在运算符之前的数学表示法。在C++中,你可以通过栈数据结构来处理逆波兰表达式的计算。以下是简单的步骤和一个简化的C++示例:
```cpp
#include <iostream>
#include <stack>
// 定义操作函数
double calculate(std::stack<double>& stack, const char op) {
double operand2 = stack.top(); stack.pop();
double operand1 = stack.top(); stack.pop();
switch (op) {
case '+':
return operand1 + operand2;
case '-':
return operand1 - operand2;
case '*':
return operand1 * operand2;
case '/':
if (operand2 != 0)
return operand1 / operand2;
else
throw "Division by zero error";
}
}
int main() {
std::string input = "2 3 + 4 *"; // 逆波兰表达式
std::istringstream iss(input);
std::stack<double> numbers;
char token;
while (iss >> token) {
if (isdigit(token)) { // 如果是数字,直接压入栈
numbers.push(stod(token));
} else { // 如果是运算符,弹出两个数并计算
double result = calculate(numbers, token);
numbers.push(result);
}
}
// 输出最终结果
std::cout << "Result: " << numbers.top() << "\n";
return 0;
}
```
在这个例子中,程序读取输入字符串,识别数字和运算符,并根据逆波兰规则逐个处理。当你运行这个程序,它会计算出"2 3 + 4 *"的结果。
阅读全文
相关推荐

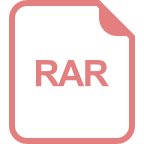
















