c++逆波兰表达式求值
时间: 2024-04-19 11:21:08 浏览: 25
C++逆波兰表达式求值是一种常见的数学表达式求值方法,也被称为后缀表达式求值。逆波兰表达式是一种不需要括号来标识优先级的表达式表示方法,它将操作符放在操作数的后面。
在C++中,可以通过使用栈来实现逆波兰表达式求值。具体步骤如下:
1. 创建一个空栈用于存储操作数。
2. 从左到右遍历逆波兰表达式的每个元素。
3. 如果当前元素是操作数,则将其压入栈中。
4. 如果当前元素是操作符,则从栈中弹出两个操作数,并根据操作符进行计算,将计算结果压入栈中。
5. 重复步骤3和步骤4,直到遍历完整个逆波兰表达式。
6. 最后,栈中剩下的唯一元素就是逆波兰表达式的求值结果。
下面是一个示例代码,用于实现逆波兰表达式求值:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <sstream>
using namespace std;
int evaluateRPN(string expression) {
stack<int> operands;
stringstream ss(expression);
string token;
while (getline(ss, token, ' ')) {
if (token == "+" || token == "-" || token == "*" || token == "/") {
int operand2 = operands.top();
operands.pop();
int operand1 = operands.top();
operands.pop();
if (token == "+") {
operands.push(operand1 + operand2);
} else if (token == "-") {
operands.push(operand1 - operand2);
} else if (token == "*") {
operands.push(operand1 * operand2);
} else if (token == "/") {
operands.push(operand1 / operand2);
}
} else {
operands.push(stoi(token));
}
}
return operands.top();
}
int main() {
string expression = "5 + 3 *";
int result = evaluatePN(expression);
cout << "Result: " << result << endl;
return 0;
}
```
以上代码中,我们使用了一个栈来存储操作数,并通过stringstream来逐个读取逆波兰表达式中的元素。如果遇到操作符,则从栈中弹出两个操作数进行计算,并将结果压入栈中。最后,栈中剩下的唯一元素就是逆波兰表达式的求值结果。
相关推荐
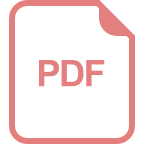















