用C++编写 利用链表实现两个多项式相乘
时间: 2024-10-20 09:12:58 浏览: 44
在C++中,我们可以使用链表数据结构来存储多项式的系数和次数,因为链表可以动态地增加或减少元素。这里我们将展示如何利用链表实现两个多项式A(x) = a0 + a1*x + ... + an*x^n 和 B(x) = b0 + b1*x + ... + bn*x^n 的逐项相乘。
首先,我们需要定义一个`Term`类,用于表示多项式的每一项:
```cpp
class Term {
public:
int coefficient; // 系数
int exponent; // 次数
Term(int c, int e) : coefficient(c), exponent(e) {}
};
```
然后,创建一个`Polynomial`类,它包含一个链表来存储多项式的项:
```cpp
class Polynomial {
private:
std::list<Term> terms;
public:
void add_term(Term term) {
terms.push_back(term);
}
// 其他操作如打印多项式...
};
```
对于两个多项式相乘,我们可以通过遍历每个多项式,并对当前项的每个幂次组合计算新的项并添加到结果多项式中。这个过程称为“Kronrod-S幂数法”或“乘积展开”。
```cpp
Polynomial multiply(Polynomial& poly1, Polynomial& poly2) {
Polynomial result;
for (const auto& term1 : poly1.terms) {
for (const auto& term2 : poly2.terms) {
int combinedExponent = term1.exponent + term2.exponent;
if (combinedExponent <= MAX_EXPONENT) { // 设置一个合理的最大指数限制
result.add_term({term1.coefficient * term2.coefficient, combinedExponent});
}
}
}
return result;
}
```
阅读全文
相关推荐
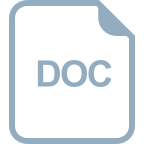
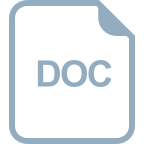
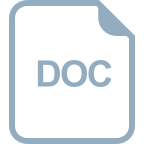

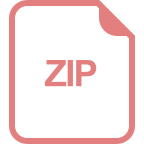
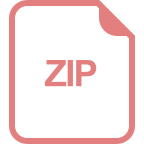
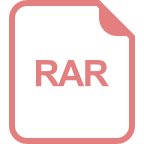
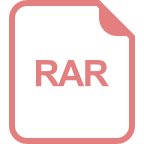
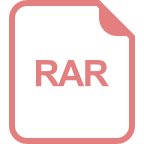
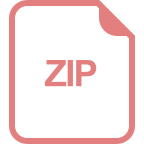






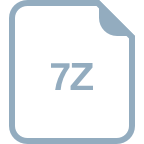