使用python编写一个脚本,要求能注释指定内容并去掉重复行
时间: 2024-12-21 13:23:15 浏览: 0
当你想要编写Python脚本来处理文本文件,其中包含一些需要注释掉的内容,并去除重复行,你可以这样做:
```python
# 导入必要的模块
import re # 用于正则表达式操作
from itertools import takewhile # 用于获取序列的唯一元素
def comment_out_lines(filename, pattern):
"""
读取文件,对匹配pattern的行添加注释并保存到新的文件中。
"""
with open(filename, 'r') as f_in, open(filename + '.commented', 'w') as f_out:
for line in f_in:
if not pattern.match(line): # 如果行不匹配模式,则原样写入
f_out.write(line)
else: # 匹配到的行前添加注释符(如#)
f_out.write('# ') + line
def remove_duplicates(filename):
"""
从原始文件中去除重复行,保留首次出现的行,然后保存到新文件中。
"""
unique_lines = list(takewhile(lambda x: x not in seen, open(filename, 'r')))
seen = set() # 使用集合存储已经看到的行,提高查找效率
with open(filename + '.no_duplicates', 'w') as f_out:
f_out.writelines(unique_lines)
# 示例:在原始文件中注释出所有以"example"开头的行,并移除重复行
pattern = re.compile(r'^example') # 创建正则表达式,匹配以"example"开头的行
comment_out_lines('input.txt', pattern)
remove_duplicates('input.commented')
```
在这个例子中,我们首先创建了一个函数`comment_out_lines`,它会读取输入文件中的每一行,如果该行不符合给定的正则表达式模式(这里假设是行首包含 "example"),则直接写入到新的文件;如果匹配,则加上注释字符 `#`。
另一个函数 `remove_duplicates` 则使用 Python 的 `itertools.takewhile` 来逐行读取输入文件,直到遇到重复的行为止。将每个唯一的行加入到 `seen` 集合中,然后一次性写入到一个新的文件中。
运行完这个脚本后,你会发现两个新文件,分别是 `input.commented` 和 `input.no_duplicates`,分别包含了已注释和去除了重复行的内容。
阅读全文
相关推荐
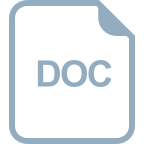
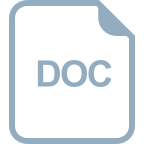
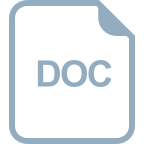
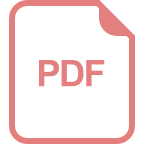


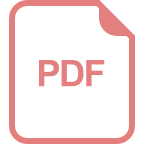
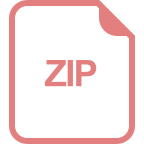
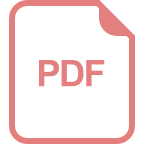
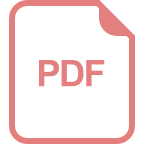
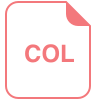






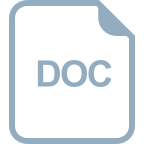
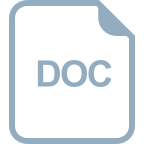
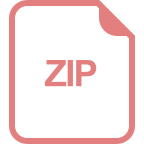