jsp学生信息管理系统idea
时间: 2025-01-01 12:33:52 浏览: 9
### JSP 学生信息管理系统 IDEA 示例教程
#### 创建 Maven 项目结构
为了构建一个高效的学生信息系统,建议采用Maven来管理依赖项。在IntelliJ IDEA中新建一个名为`StudentInfoSystem`的Maven项目。
```xml
<dependencies>
<!-- Servlet API -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
<!-- MySQL Connector -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
<!-- Other dependencies as needed... -->
</dependencies>
```
#### 配置数据库连接池
配置文件 `src/main/resources/db.properties` 中定义数据库参数:
```properties
jdbc.driver=com.mysql.cj.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/student_info?useSSL=false&serverTimezone=UTC
jdbc.username=root
jdbc.password=password
```
#### 设计实体类
创建代表学生数据模型的 Java 类,在包 `com.example.entity` 下建立 Student.java 文件[^2]。
```java
package com.example.entity;
public class Student {
private int id;
private String name;
private String gender;
private Date birthDate;
// Getters and Setters...
}
```
#### 开发 DAO 层接口及其实现
DAO (Data Access Object) 负责处理与数据库交互的任务。编写接口 IStudentDao 和其对应的 MyBatis 映射器 XML 或者 JDBC 实现版本[^4]。
```java
// src/main/java/com/example/dao/IStudentDao.java
package com.example.dao;
import java.util.List;
import com.example.entity.Student;
public interface IStudentDao {
List<Student> getAllStudents();
void addNewStudent(Student student);
boolean updateStudentById(int id, Student updatedStudent);
void deleteStudentById(int id);
}
// Implementation using plain JDBC or MyBatis mapper here.
```
#### 编写业务逻辑层 Service
Service 组件负责协调各个组件之间的操作流程并执行必要的验证工作。新增服务类 StudentServiceImpl 来封装具体的服务方法。
```java
@Service
public class StudentServiceImpl implements IStudentService {
@Autowired
private IStudentDao dao;
public List<Student> fetchAll() { ... }
public void saveOrUpdate(Student s) { ... }
public void remove(Integer sid) { ... }
}
```
#### 构建控制器 Controller 处理 HTTP 请求
Controller 接收来自用户的请求并将响应返回给前端页面。这里展示如何设置 RESTful 风格的端点以供浏览器调用[^5]。
```java
@RestController
@RequestMapping("/api/students")
public class StudentController {
@GetMapping("")
public ResponseEntity<List<Student>> listAll(@RequestParam(value="page", defaultValue="1") Integer page,
@RequestParam(value="size", defaultValue="10") Integer size){
Pageable pageable = PageRequest.of(page - 1, size);
return new ResponseEntity<>(service.fetchAll(), HttpStatus.OK);
}
// More endpoints like POST / PUT / DELETE ...
}
```
#### 前端视图设计
最后一步是准备用于显示和编辑学生的 HTML 页面以及样式表单。可以考虑引入 Bootstrap 库简化界面布局过程[^3]。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8"/>
<title>Student Information System</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"/>
</head>
<body>
<div class="container mt-5">
<h1 class="text-center">Manage Students</h1>
<table class="table table-bordered">
<thead><tr><th>ID</th><th>Name</th><th>Gender</th><th>Date of Birth</th></tr></thead>
<tbody>
<!-- Use JSTL tags to iterate over the students collection -->
<c:forEach var="student" items="${students}">
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
<td>${student.gender}</td>
<td>${student.birthDate}</td>
</tr>
</c:forEach>
</tbody>
</table>
</div>
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.9.2/dist/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script>
</body>
</html>
```
阅读全文
相关推荐



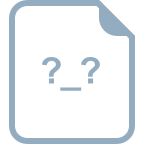







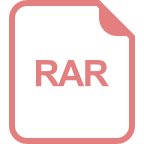






