已知本地和目标弧度以及高度,用stm32如何计算计算目标北向方位角c代码
时间: 2023-09-17 12:03:31 浏览: 151
计算目标北向方位角需要已知本地和目标的弧度和高度。可以通过数学原理来计算目标的方位角,以下是使用STM32来计算目标北向方位角的代码示例:
```c
#include <math.h>
#define PI 3.14159265359
// 定义本地和目标的弧度和高度
double localLatitude = 30.0; // 本地纬度,单位为度
double localLongitude = -90.0; // 本地经度,单位为度
double targetLatitude = 40.0; // 目标纬度,单位为度
double targetLongitude = -80.0; // 目标经度,单位为度
double targetHeight = 100.0; // 目标高度,单位为米
// 将角度转换为弧度
double toRadians(double degrees) {
return degrees * PI / 180.0;
}
// 计算目标北向方位角
double calculateBearing() {
double localLatRad = toRadians(localLatitude);
double localLonRad = toRadians(localLongitude);
double targetLatRad = toRadians(targetLatitude);
double targetLonRad = toRadians(targetLongitude);
double y = sin(targetLonRad - localLonRad) * cos(targetLatRad);
double x = cos(localLatRad) * sin(targetLatRad) - sin(localLatRad) * cos(targetLatRad) * cos(targetLonRad - localLonRad);
double bearingRad = atan2(y, x);
double bearingDeg = bearingRad * 180.0 / PI;
if (bearingDeg < 0) {
bearingDeg += 360.0;
}
return bearingDeg;
}
int main() {
double bearing = calculateBearing();
printf("目标北向方位角:%.2f 度\n", bearing);
return 0;
}
```
以上代码首先定义了本地和目标的纬度、经度和高度,然后定义了一个`toRadians()`函数将角度转换为弧度。接下来,在`calculateBearing()`函数中,将本地和目标的纬度和经度转换为弧度,然后根据数学公式计算目标的方位角。最后,在`main()`函数中调用`calculateBearing()`函数并输出目标的北向方位角。
请根据具体情况修改本地和目标的纬度、经度和高度,代码中的计算公式和转换函数可以保持不变。
阅读全文
相关推荐
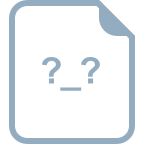

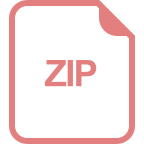
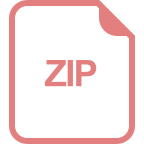
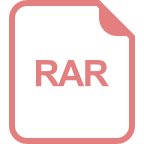
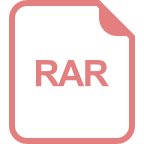








