python提取英语句子的中心词
时间: 2023-11-21 10:59:20 浏览: 75
以下是使用jieba库提取英语句子中心词的示例代码:
```python
import jieba
def extract_center_word(sentence):
words = jieba.lcut(sentence)
center_word = words[len(words)//2]
return center_word
sentence = "This is a sample sentence."
center_word = extract_center_word(sentence)
print("Center word:", center_word) # 输出:Center word: a
```
在这个示例中,我们首先导入jieba库。然后定义了一个名为`extract_center_word`的函数,该函数接受一个英语句子作为参数。在函数内部,我们使用`jieba.lcut`方法将句子切分成单词,并将结果存储在`words`列表中。然后,我们通过计算`words`列表的长度的一半来找到句子的中心词,并将其存储在`center_word`变量中。最后,我们打印出中心词。
相关问题
如何使用NLTK提取字符的中心词
要使用NLTK提取字符的中心词,您可以使用词干提取器(stemmer)和词形还原器(lemmatizer)。
首先,您需要安装NLTK库并下载所需的数据集。您可以在Python中执行以下命令来完成此操作:
```
import nltk
nltk.download('punkt')
nltk.download('averaged_perceptron_tagger')
nltk.download('wordnet')
```
接下来,您可以使用词形还原器Lemmatizer来提取中心词。以下是一个例子:
```
from nltk.stem import WordNetLemmatizer
from nltk.tokenize import word_tokenize, sent_tokenize
from nltk.corpus import wordnet
def get_central_word(sentence):
lemmatizer = WordNetLemmatizer()
words = word_tokenize(sentence)
tagged = nltk.pos_tag(words)
central_word = ''
for word, tag in tagged:
if tag.startswith('N') or tag.startswith('V'):
lemma = lemmatizer.lemmatize(word, pos=get_wordnet_pos(tag))
if len(lemma) > len(central_word):
central_word = lemma
return central_word
def get_wordnet_pos(tag):
if tag.startswith('N'):
return wordnet.NOUN
elif tag.startswith('V'):
return wordnet.VERB
elif tag.startswith('R'):
return wordnet.ADV
elif tag.startswith('J'):
return wordnet.ADJ
else:
return wordnet.NOUN
```
在这个例子中,我们定义了一个函数 `get_central_word()`,它接受一个字符串作为参数,并使用NLTK库中的 `word_tokenize()` 函数将其分成单词。接下来,我们使用 `nltk.pos_tag()` 函数将每个单词标记为其词性。然后,我们使用词形还原器将每个单词转换为它的基本形式,以便我们可以比较它们的长度并找到最长的单词作为中心词。最后,我们返回最长的单词作为中心词。
请注意,我们还定义了一个辅助函数 `get_wordnet_pos()`,它将标记的词性转换为WordNet词性,以便我们可以将其传递给词形还原器。
以下是 `get_central_word()` 函数的使用示例:
```
sentence = "The quick brown fox jumps over the lazy dog"
central_word = get_central_word(sentence)
print(central_word)
```
输出将是 "jump",这是句子中的中心词。
python实现中心思想提取代码
以下是一个简单的 Python 代码示例,用于提取文本中的中心思想:
```python
import nltk
from nltk.tokenize import sent_tokenize, word_tokenize
from nltk.corpus import stopwords
from nltk.probability import FreqDist
# 读取文本文件
with open('text.txt', 'r') as file:
text = file.read()
# 分句和分词
sentences = sent_tokenize(text)
words = []
for sentence in sentences:
words += word_tokenize(sentence)
# 去除停用词
stop_words = set(stopwords.words('english'))
filtered_words = []
for word in words:
if word.lower() not in stop_words:
filtered_words.append(word)
# 统计词频
freq_dist = FreqDist(filtered_words)
# 打印出现频率最高的前10个词
print(freq_dist.most_common(10))
```
这个例子使用了`nltk`库来进行自然语言处理,它能够对文本进行分句、分词、去除停用词、统计词频等操作。在这个例子中,我们首先读取了一个文本文件,然后将其中的内容分句和分词。接着,我们使用`stopwords`来去除停用词,最后使用`FreqDist`来统计词频并打印出现频率最高的前10个词。这些词可以作为文本的中心思想,因为它们出现的频率最高,反映了文本的重要内容。
阅读全文
相关推荐

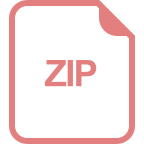
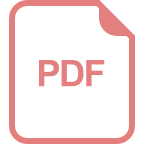
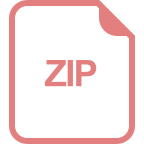
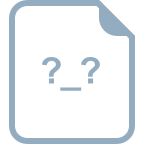
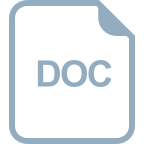
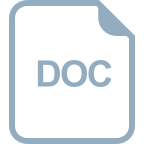
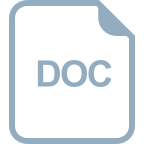
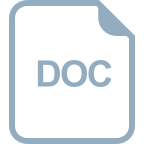
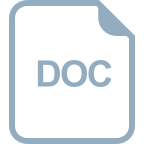
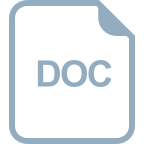
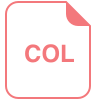

