java 保存 buffer到本地
时间: 2024-09-14 22:06:50 浏览: 32
在Java中,将Buffer保存到本地通常指的是将存储在Buffer中的数据写入到磁盘的文件中。这可以通过使用`java.nio.file`包中的类来实现,如`Path`和`Files`类,结合使用`FileChannel`。以下是一个简单的例子来说明这个过程:
```java
import java.io.BufferedOutputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
public class BufferToFileExample {
public static void main(String[] args) {
// 创建一个ByteBuffer,填充数据
ByteBuffer buffer = ByteBuffer.allocate(1024);
// 假设已经有一些数据写入到buffer中...
// 定义文件路径
Path path = Paths.get("example.bin");
try (FileOutputStream fos = new FileOutputStream(path.toString());
BufferedOutputStream bos = new BufferedOutputStream(fos);
FileChannel fileChannel = fos.getChannel()) {
// 将文件通道定位到文件的开头
fileChannel.position(0);
// 将buffer中的数据写入到文件中
while (buffer.hasRemaining()) {
fileChannel.write(buffer);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上面的例子中,我们首先创建了一个`ByteBuffer`对象并填充了数据。接着定义了要写入数据的文件路径,使用`FileOutputStream`和`FileChannel`将数据写入到这个文件中。这里使用了try-with-resources语句来自动关闭流,以保证资源的正确释放。
此外,`FileChannel`还提供了更灵活的文件操作选项,比如追加数据到文件末尾、创建新文件、读取和写入等。当使用`Files`类的方法时,也可以用类似的方式实现相同的功能,但通常更为简洁。
阅读全文
相关推荐
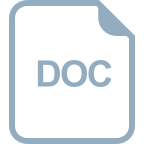
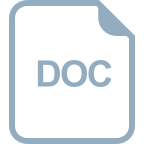
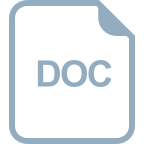

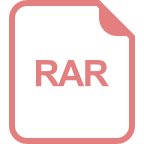













