ros消息发布订阅学生学号
时间: 2024-11-05 10:29:54 浏览: 20
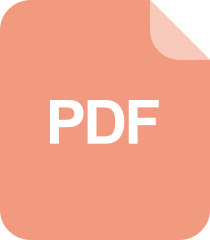
Introduction to ROS Publisher-Subscriber Nodes
ROS (Robot Operating System) 是一种广泛用于机器人系统开发的中间件,它支持节点间的消息传递。在ROS中,如果你需要创建一个关于“学生学号”的消息,首先你需要定义一个自定义的数据类型,比如`std_msgs/String`或者自定义一个包含学号信息的`msg`包。
1. 定义消息类型:你可以创建一个新的`msg`包,并在其中定义一个`StudentID`.msg文件,内容可能是这样的:
```cpp
message StudentID {
string id = 1; // 学生学号字段
}
```
2. 发布者和订阅者:发布者(`Publisher`)负责发送`StudentID`消息,订阅者(`Subscriber`)则接收并处理这些消息。例如,在发布者的部分,你会实例化一个`Publisher`,并将学生的学号作为参数发布出去:
```c++
#include <ros/ros.h>
#include "msg/StudentID.h"
void publish_student_id(const std::string& student_id) {
ros::NodeHandle nh;
ros::Publisher pub = nh.advertise<msg::StudentID>("student_id_topic", 10); // 设置主题名
msg::StudentID msg;
msg.id = student_id;
pub.publish(msg);
}
int main(int argc, char** argv) {
ros::init(argc, argv, "student_id_publisher");
publish_student_id("123456");
// ...
return 0;
}
```
而在订阅者部分,你需要创建一个回调函数来处理接收到的消息:
```c++
#include <ros/ros.h>
#include "msg/StudentID.h"
void on_student_id_received(const msg::StudentID::ConstPtr& msg) {
std::cout << "Received student ID: " << msg->id << std::endl;
}
int main(int argc, char** argv) {
ros::init(argc, argv, "student_id_subscriber");
ros::Subscriber sub = nh.subscribe("student_id_topic", 1, &on_student_id_received); // 订阅主题
// ...
ros::spin();
return 0;
}
```
阅读全文
相关推荐
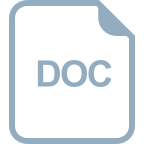
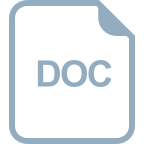
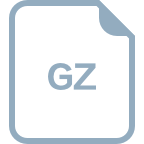
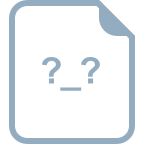
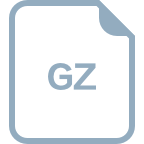
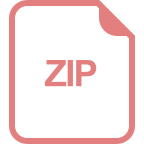
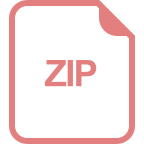
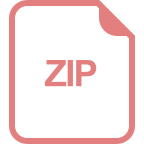
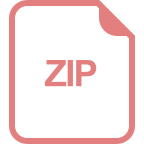
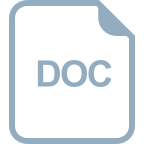
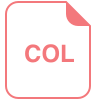
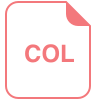
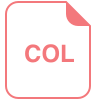


