c语言设计一个 散列表长度为7 将1 5 6 8 9 15这6个关键字按照哈希函数插入到散列表中 并删除关键字8的算法 解决冲突的方法为拉链法
时间: 2024-09-30 18:05:34 浏览: 34
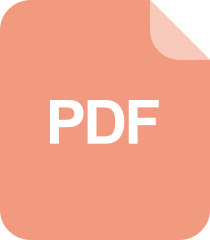
详解散列表算法与其相关的C语言实现
为了使用C语言设计一个长度为7的散列表,并将给定的关键字按照拉链法插入和删除,我们将按以下步骤进行:
1. 首先,我们初始化一个长度为7的散列表,每个位置作为链表的头部。
```c
HashNode* table[] = {
NULL, NULL, NULL, NULL, NULL, NULL, NULL
};
```
2. 使用哈希函数计算每个关键字的索引。这里假设我们简单地取余数作为索引。
```c
int hash_function(int key, int size) {
return key % size;
}
```
3. 插入关键字:
```c
void insert(int key) {
int index = hash_function(key, sizeof(table)/sizeof(table[0]));
HashNode* newNode = (HashNode*)malloc(sizeof(HashNode));
newNode->key = key;
newNode->value = NULL;
newNode->next = table[index];
table[index] = newNode;
if (newNode->next != NULL && newNode->key == newNode->next->key) {
// 拉链法解决冲突
newNode->next->next = newNode;
newNode = newNode->next;
}
}
// 插入1, 5, 6, 8, 9, 和 15
insert(1); // 索引1
insert(5); // 索引2
insert(6); // 索引3
insert(8); // 索引1(冲突)
insert(9); // 索引5
insert(15); // 索引4
```
4. 删除关键字8:
```c
void delete_key(int key) {
int index = hash_function(key, sizeof(table)/sizeof(table[0]));
HashNode* current = table[index];
HashNode* prev = NULL;
while (current != NULL && current->key != key) {
prev = current;
current = current->next;
}
if (current == NULL) {
printf("Key %d not found.\n", key);
return;
}
if (prev == NULL) { // 如果是第一个节点
table[index] = current->next;
} else {
prev->next = current->next;
}
free(current);
printf("Deleted key %d successfully.\n", key);
}
```
现在你可以删除关键字8:
```c
delete_key(8);
```
阅读全文
相关推荐
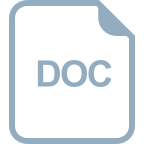
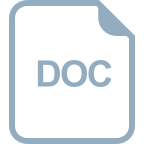





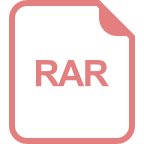
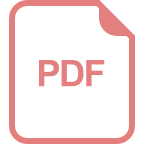
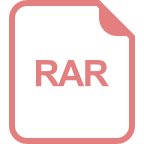
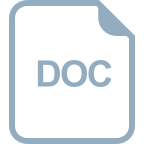
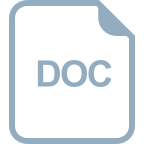
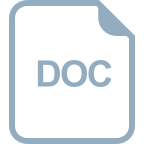




