写一个C++的代码,删除字符串中某个下标到另一个下标之间的字符并将剩余内容保存到新的字符串中
时间: 2024-10-24 16:12:14 浏览: 17
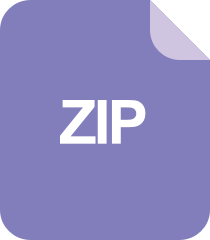
c++-c++编程基础之leetcode题解第28题找出字符串第一个匹配项的下标.zip
在C++中,你可以使用`std::string`的`substr()`和`sresize()`函数来实现这个功能。下面是一个简单的例子,假设我们有一个名为`source_string`的原始字符串,并想删除从索引`start_index`到`end_index`之间的所有字符,并将结果保存在`new_string`中:
```cpp
#include <iostream>
#include <string>
std::string removeSubstring(const std::string& source_string, int start_index, int end_index) {
if (start_index < 0 || end_index > source_string.length() || start_index > end_index) {
std::cerr << "Invalid indices!" << std::endl;
return "";
}
// 获取待保留部分的子串
std::string new_substring = source_string.substr(0, start_index) + source_string.substr(end_index + 1);
// 调整新字符串长度
new_substring.resize(new_substring.size());
return new_substring;
}
int main() {
std::string source_string = "Hello, World!";
int start_index = 7;
int end_index = 11; // 注意这里end_index并不包含在内
std::string new_string = removeSubstring(source_string, start_index, end_index);
if (!new_string.empty()) {
std::cout << "New string after removing characters from index " << start_index << " to " << end_index << ": " << new_string << std::endl;
}
return 0;
}
```
在这个例子中,如果提供的起始和结束索引超出范围,程序会打印错误消息并返回空字符串。然后它创建一个新的字符串,只保留了开始和结束索引之外的部分。
阅读全文
相关推荐
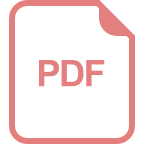
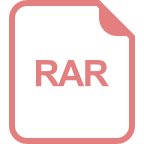















