vscode编写python如何导入csv文件
时间: 2024-10-07 11:05:00 浏览: 63
在Visual Studio Code (VSCode) 中编写Python程序并导入CSV文件通常涉及以下步骤:
1. 安装所需库:首先,你需要安装`pandas`库,它提供了一个方便的方式来处理CSV文件。如果还未安装,可以在终端或命令提示符里运行以下命令(如果你还没有安装pip,需要先安装):
```
!pip install pandas
```
2. 导入模块:在Python代码中,你需要导入`pandas`模块来读取CSV文件。你可以这样做:
```python
import pandas as pd
```
3. 读取CSV文件:使用`pd.read_csv()`函数打开CSV文件,例如你的文件名为"data.csv",位置在当前工作目录下,代码会是这样:
```python
data = pd.read_csv("data.csv")
```
4. 检查数据:读取后,你可以查看数据前几行或者整体信息:
```python
print(data.head())
```
5. 使用数据:现在你可以对数据进行操作、分析或保存到其他格式。
相关问题
刚刚安装Vscode和python,请在一个全新空白的系统中写一段简单的线性回归分析代码,保证成功运行
在Visual Studio Code (VSCode) 中编写一个简单的Python线性回归分析示例,我们可以使用内置的数据集以及scikit-learn库,这是一个非常流行用于机器学习的Python库。首先确保已经安装了必要的库,包括`pandas`, `matplotlib`, 和 `sklearn`。如果你还没有安装,可以在终端或命令提示符中使用`pip install pandas matplotlib sklearn`命令。
下面是一个基础的线性回归模型的例子:
```python
# 导入所需的库
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
import matplotlib.pyplot as plt
# 加载数据(这里我们使用随机生成的数据,实际应用中可能需要从文件或API获取)
# 这里假设有一个名为'data.csv'的CSV文件,包含两列数据 'feature' 和 'target'
df = pd.read_csv('data.csv')
# 分割特征和目标变量
X = df['feature'].values.reshape(-1, 1)
y = df['target'].values.reshape(-1, 1)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
# 创建线性回归模型
model = LinearRegression()
# 训练模型
model.fit(X_train, y_train)
# 预测
y_pred = model.predict(X_test)
# 可视化结果
plt.scatter(X_test, y_test, color='blue')
plt.plot(X_test, y_pred, color='red', linewidth=3)
plt.xlabel('Feature')
plt.ylabel('Target')
plt.title('Linear Regression')
plt.show()
```
保存这段代码到`main.py`(或其他你喜欢的文件名),然后在VSCode中打开,点击左下角的"Run Python File in Terminal"按钮,或者按F5运行。确保数据文件路径正确,并且你的环境能够找到所需库。
在VScode上要用Python进行数据挖掘,实现对花卉的分类,需要用到那些工具,配置那些环境
在Visual Studio Code (VSCode) 上进行Python数据挖掘并针对花卉数据进行分类,你需要准备以下工具和设置:
1. **基本安装**:
- 安装 **Python**:首先确保你的电脑已经安装了Python,推荐使用最新版本(如Python 3.7或更高),可以从官网下载安装 https://www.python.org/downloads/。
- **VSCode**:安装VSCode本身,它是轻量级且功能强大的代码编辑器,可以从官方下载 https://code.visualstudio.com/download。
2. **数据处理库**:
- **Pandas**: 数据清洗、预处理和分析工具,可以用于加载、操作和分析花卉数据。安装命令:`pip install pandas`
- **NumPy**: 数值计算库,用于处理大量数值数据。安装命令:`pip install numpy`
- **Matplotlib** 或 **Seaborn**: 可视化库,帮助绘制图表展示数据特征。安装命令:`pip install matplotlib seaborn`
3. **机器学习库**:
- **Scikit-learn**: 主流的机器学习库,包含许多分类算法,如决策树、随机森林等。安装命令:`pip install scikit-learn`
- **TensorFlow** 或 **Keras**: 如果你计划使用深度学习,这两个库是首选,它们支持神经网络模型。安装命令分别:`pip install tensorflow` 和 `pip install keras`(如果选择Keras,记得先安装TensorFlow)
4. **环境配置**:
- 确保你有一个虚拟环境(Virtual Environment)管理项目依赖,这有助于避免全局包之间的冲突。可以在终端或VSCode中通过`venv`模块创建,例如:
```sh
python - Windows: `.\my_flower_classifier\Scripts\activate`
- Linux/MacOS: `source my_flower_classifier/bin/activate`
- 在激活的环境中安装所需的库,例如`pip install -r requirements.txt`(如果有requirements.txt文件列出所有依赖)
5. **数据集**:
- 获取花卉数据集,比如Flowers102、Oxford 102 Flowers等,可以在线找到并下载到本地。将数据集放在项目文件夹内,并熟悉其结构和标签。
在配置好以上环境后,你可以开始编写代码,包括数据加载、预处理、特征工程、模型训练和评估。以下是简单的步骤示例:
```python
# 导入库
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
# 加载数据
data = pd.read_csv('flowers.csv')
# 数据预处理...
X = data.drop('species', axis=1)
y = data['species']
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 特征缩放
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
# 训练模型
clf = RandomForestClassifier()
clf.fit(X_train, y_train)
# 预测和评估
y_pred = clf.predict(X_test)
print(classification_report(y_test, y_pred))
```
最后别忘了验证模型效果,调整参数,以及可视化结果以便于理解和优化。
阅读全文
相关推荐
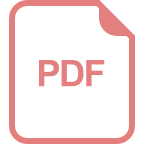
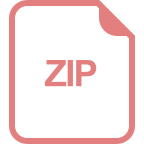
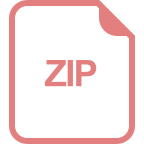
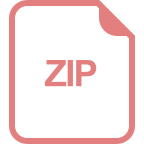
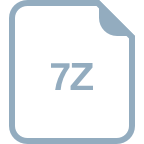
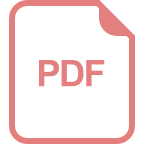
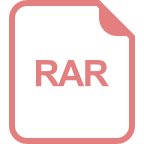
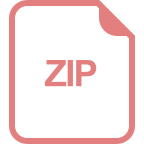
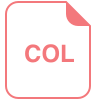
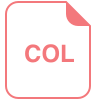
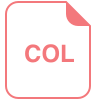
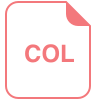
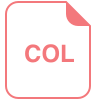
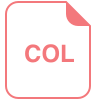



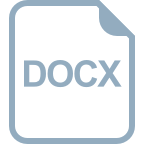