用c语言写一个商品价格计算的代码
时间: 2024-09-24 08:26:24 浏览: 41
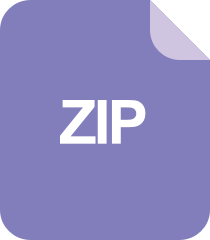
C语言代码做的商品管理系统.zip
当然可以。在C语言中,我们可以编写一个简单的程序来计算商品价格,假设我们有一个基本的结构体表示商品,包含名称和单价,然后我们可以创建函数来处理加价、减价等操作。这是一个简单的例子:
```c
#include <stdio.h>
// 定义商品结构体
typedef struct {
char name[50];
float price;
} Product;
// 计算价格的函数,这里仅用于演示加法,可以根据需求增加更多操作
float calculate_price(Product product, int operation, float amount) {
switch (operation) {
case 1: // 加价
return product.price + amount;
case 2: // 减价
if (amount > 0) { // 防止负数折扣
return product.price - amount;
} else {
printf("Invalid discount amount.\n");
return product.price; // 如果输入负数,保持原价
}
default:
printf("Unsupported operation.\n");
return product.price;
}
}
int main() {
Product item = {"牛奶", 20.0};
float new_price, discount_amount = 5.0;
printf("Original price of %s is %.2f.\n", item.name, item.price);
// 示例加价和减价
new_price = calculate_price(item, 1, discount_amount);
printf("After adding a discount of %.2f, the new price is %.2f.\n", discount_amount, new_price);
new_price = calculate_price(item, 2, discount_amount);
printf("After applying a discount of %.2f, the new price is %.2f.\n", discount_amount, new_price);
return 0;
}
```
阅读全文
相关推荐
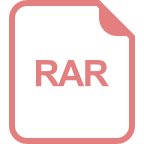
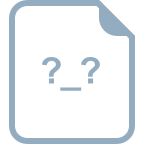




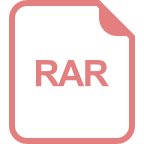
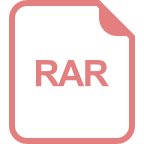
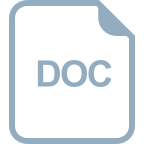
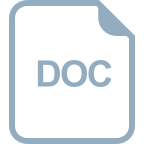
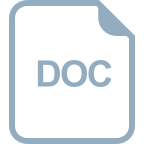






