用c语言写一个文具销售管理系统能够完成实现信息录入,在已有信息基础上添加新的记录,删除、修改指定商品编号记录,能够按编号查寻设备,计算当日销售额并注明代码信息
时间: 2024-04-14 10:30:49 浏览: 36
当然可以!下面是一个简单的示例代码,演示了如何实现您提到的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Product {
int id;
char name[50];
float price;
};
struct SalesRecord {
int productId;
int quantity;
};
struct Product products[100];
struct SalesRecord sales[100];
int productCount = 0;
int salesCount = 0;
void addProduct() {
printf("请输入商品编号: ");
scanf("%d", &products[productCount].id);
printf("请输入商品名称: ");
scanf("%s", products[productCount].name);
printf("请输入商品价格: ");
scanf("%f", &products[productCount].price);
productCount++;
printf("商品添加成功!\n");
}
void addSales() {
printf("请输入销售的商品编号: ");
int productId;
scanf("%d", &productId);
printf("请输入销售数量: ");
int quantity;
scanf("%d", &quantity);
// 查找商品
int found = 0;
for (int i = 0; i < productCount; i++) {
if (products[i].id == productId) {
found = 1;
break;
}
}
if (found) {
sales[salesCount].productId = productId;
sales[salesCount].quantity = quantity;
salesCount++;
printf("销售记录添加成功!\n");
} else {
printf("找不到指定的商品编号!\n");
}
}
void deleteProduct() {
printf("请输入要删除的商品编号: ");
int productId;
scanf("%d", &productId);
int found = 0;
for (int i = 0; i < productCount; i++) {
if (products[i].id == productId) {
// 删除商品
for (int j = i; j < productCount - 1; j++) {
products[j] = products[j + 1];
}
productCount--;
found = 1;
printf("商品删除成功!\n");
break;
}
}
if (!found) {
printf("找不到指定的商品编号!\n");
}
}
void modifyProduct() {
printf("请输入要修改的商品编号: ");
int productId;
scanf("%d", &productId);
int found = 0;
for (int i = 0; i < productCount; i++) {
if (products[i].id == productId) {
printf("请输入新的商品名称: ");
scanf("%s", products[i].name);
printf("请输入新的商品价格: ");
scanf("%f", &products[i].price);
found = 1;
printf("商品修改成功!\n");
break;
}
}
if (!found) {
printf("找不到指定的商品编号!\n");
}
}
void searchProduct() {
printf("请输入要查找的商品编号: ");
int productId;
scanf("%d", &productId);
int found = 0;
for (int i = 0; i < productCount; i++) {
if (products[i].id == productId) {
printf("商品编号: %d\n", products[i].id);
printf("商品名称: %s\n", products[i].name);
printf("商品价格: %.2f\n", products[i].price);
found = 1;
break;
}
}
if (!found) {
printf("找不到指定的商品编号!\n");
}
}
void calculateDailySales() {
float totalSales = 0.0;
for (int i = 0; i < salesCount; i++) {
int productId = sales[i].productId;
int quantity = sales[i].quantity;
// 查找商品
for (int j = 0; j < productCount; j++) {
if (products[j].id == productId) {
float price = products[j].price;
totalSales += price * quantity;
break;
}
}
}
printf("当日销售额: %.2f\n", totalSales);
}
int main() {
int choice;
do {
printf("\n********** 文具销售管理系统 **********\n");
printf("1. 添加商品\n");
printf("2. 添加销售记录\n");
printf("3. 删除商品\n");
printf("4. 修改商品信息\n");
printf("5. 查找商品\n");
printf("6. 计算当日销售额\n");
printf("0. 退出系统\n");
printf("************************************\n");
printf("请输入操作选项: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addProduct();
break;
case 2:
addSales();
break;
case 3:
deleteProduct();
break;
case 4:
modifyProduct();
break;
case 5:
searchProduct();
break;
case 6:
calculateDailySales();
break;
case 0:
printf("感谢使用文具销售管理系统!\n");
break;
default:
printf("无效的选项,请重新输入!\n");
break;
}
} while (choice != 0);
return 0;
}
```
这只是一个简单的示例,您可以根据实际需求进行扩展和修改。希望对您有所帮助!
相关推荐
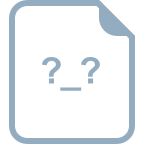
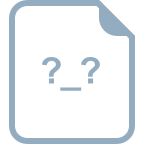
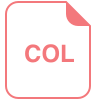
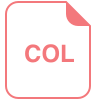
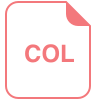
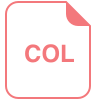
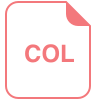









