飞翔的小鸟python
时间: 2023-12-05 09:40:49 浏览: 45
以下是一个简单的飞翔的小鸟Python游戏的实现,包括小鸟和管道的类方法:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 288
screen_height = 512
screen = pygame.display.set_mode((screen_width, screen_height))
# 加载背景图片
background = pygame.image.load("background.png").convert()
# 加载地面图片
ground = pygame.image.load("ground.png").convert()
# 加载小鸟图片
bird_images = [
pygame.image.load("bird1.png").convert_alpha(),
pygame.image.load("bird2.png").convert_alpha(),
pygame.image.load("bird3.png").convert_alpha()
]
# 加载管道图片
pipe_image = pygame.image.load("pipe.png").convert_alpha()
# 设置字体
font = pygame.font.SysFont(None, 48)
# 定义小鸟类
class Bird(pygame.sprite.Sprite):
def __init__(self, x, y):
pygame.sprite.Sprite.__init__(self)
self.images = bird_images
self.index = 0
self.image = self.images[self.index]
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
self.speed = 0
self.gravity = 0.5
def update(self, flap=False):
if flap:
self.speed = -10
else:
self.speed += self.gravity
self.rect.y += self.speed
self.index += 1
if self.index >= len(self.images):
self.index = 0
self.image = self.images[self.index]
def go_die(self):
self.speed = 0
self.gravity = 0
self.images = [pygame.image.load("bird_die.png").convert_alpha()]
# 定义管道类
class Pipe(pygame.sprite.Sprite):
def __init__(self, x, y, upwards=True):
pygame.sprite.Sprite.__init__(self)
self.image = pipe_image
self.rect = self.image.get_rect()
self.rect.x = x
if upwards:
self.image = pygame.transform.flip(self.image, False, True)
self.rect.y = y - self.rect.height - 100
else:
self.rect.y = y + 100
def update(self):
self.rect.x -= 5
# 创建小鸟和管道的精灵组
bird_group = pygame.sprite.Group()
pipe_group = pygame.sprite.Group()
# 创建小鸟对象并添加到精灵组
bird = Bird(50, 200)
bird_group.add(bird)
# 游戏主循环
clock = pygame.time.Clock()
score = 0
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bird.update(flap=True)
# 更新小鸟和管道
bird.update()
pipe_group.update()
# 检测小鸟是否碰到地面或者管道
if pygame.sprite.spritecollide(bird, pipe_group, False) or bird.rect.bottom >= screen_height - ground.get_height():
bird.go_die()
# 检测小鸟是否通过管道
for pipe in pipe_group:
if pipe.rect.right < bird.rect.left and not pipe.passed:
pipe.passed = True
score += 1
# 添加新的管道
if len(pipe_group) < 3:
pipe = Pipe(screen_width, random.randint(100, 400))
pipe_group.add(pipe)
# 绘制游戏界面
screen.blit(background, (0, 0))
pipe_group.draw(screen)
bird_group.draw(screen)
screen.blit(ground, (0, screen_height - ground.get_height()))
# 显示分数
score_text = font.render(str(score), True, (255, 255, 255))
screen.blit(score_text, (screen_width // 2 - score_text.get_width() // 2, 50))
# 更新屏幕
pygame.display.update()
# 控制游戏帧率
clock.tick(30)
```
相关推荐
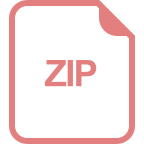














