A*算法求解TSP python
时间: 2024-10-15 12:02:57 浏览: 8
A*算法是一种启发式搜索算法,常用于解决旅行商问题(Traveling Salesman Problem, TSP),特别是在寻找最短路径或最少成本路径时。在Python中,我们可以利用这种算法来寻找给定城市间的最优化路线。
首先,你需要安装一些必要的库,如`networkx`用于图的操作,`heapq`用于优先队列:
```bash
pip install networkx
```
下面是一个简单的A*算法求解TSP问题的Python实现示例:
```python
import heapq
import networkx as nx
def heuristic(a, b):
# 计算两点之间的曼哈顿距离或其他合适的启发式函数
x1, y1 = a
x2, y2 = b
return abs(x1 - x2) + abs(y1 - y2)
def astar_tsp(graph, start, goal):
closed_set = set()
open_set = [(0, start)]
while open_set:
current_distance, current_node = heapq.heappop(open_set)
if current_node not in closed_set:
if current_node == goal:
path = [current_node]
parent = current_node
while parent != start:
parent = graph[parent][0] # 使用字典形式的邻接表
path.append(parent)
return list(reversed(path)) # 返回从起点到终点的路径
closed_set.add(current_node)
for neighbor, distance in graph[current_node].items():
if neighbor not in closed_set:
new_distance = current_distance + distance
priority = new_distance + heuristic(neighbor, goal)
if neighbor not in open_set or priority < open_set[open_set.index((priority, neighbor))]:
graph[current_node][neighbor]['distance'] = new_distance # 更新邻居节点的距离信息
heapq.heappush(open_set, (priority, neighbor))
raise ValueError("No solution found")
# 创建一个带权重的城市图(例如,曼哈顿距离作为权重)
graph = nx.Graph()
cities = ... # 城市列表,每个元素表示一个节点,包含坐标等信息
for city in cities:
graph.add_node(city)
# 添加边及权重(假设cost是从一个城市到另一个城市的直接距离)
for i in range(len(cities)):
for j in range(i+1, len(cities)):
cost = heuristic(cities[i], cities[j])
graph.add_edge(cities[i], cities[j], weight=cost)
start_city = cities[0] # 设置起始城市
solution_path = astar_tsp(graph, start_city, start_city) # 因为TSP通常需要返回环路,这里从起点出发回到起点
print(solution_path)
```
阅读全文
相关推荐
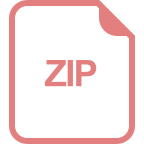
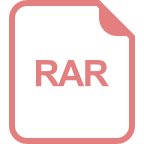
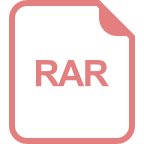
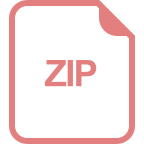
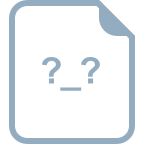
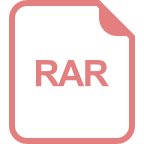












