python蚁群算法求解tsp
时间: 2023-08-25 07:07:10 浏览: 157
蚁群算法(Ant Colony Optimization,简称ACO)是一种基于模拟蚂蚁觅食行为的优化算法,常用于求解旅行商问题(Traveling Salesman Problem,简称TSP)。下面是使用Python实现蚁群算法求解TSP的基本步骤:
1. 定义TSP问题的输入,包括城市数量、城市间距离矩阵等。
2. 初始化蚂蚁群和相关参数,包括蚂蚁数量、信息素浓度、信息素挥发率、启发式因子等。
3. 对每只蚂蚁进行路径选择和更新。
- 蚂蚁按照一定的规则选择下一个要访问的城市,可以利用信息素浓度和启发式因子来决定选择概率。
- 每只蚂蚁完成一次路径选择后,更新路径长度和信息素浓度。
4. 更新全局最优路径。
- 在所有蚂蚁完成路径选择后,根据各个路径长度更新全局最优路径。
5. 更新信息素浓度。
- 通过信息素挥发和新的信息素分泌来更新每条路径上的信息素浓度。
6. 重复步骤3-5,直到满足停止条件(如迭代次数达到限制或找到较优解)。
下面是一个简单的Python代码示例:
```python
import numpy as np
def ant_colony_optimization(cities, num_ants, num_iterations, evaporation_rate, alpha, beta):
num_cities = len(cities)
pheromone = np.ones((num_cities, num_cities)) # 初始化信息素浓度矩阵
best_path = None
best_length = float('inf')
for _ in range(num_iterations):
paths = []
lengths = []
for _ in range(num_ants):
path = []
length = 0
current_city = np.random.randint(num_cities)
unvisited_cities = set(range(num_cities))
unvisited_cities.remove(current_city)
while unvisited_cities:
next_city = select_next_city(current_city, unvisited_cities, pheromone, alpha, beta)
path.append(next_city)
length += cities[current_city][next_city]
current_city = next_city
unvisited_cities.remove(current_city)
path.append(path[0]) # 回到起始城市
length += cities[current_city][path[0]]
paths.append(path)
lengths.append(length)
if length < best_length:
best_path = path
best_length = length
update_pheromone(pheromone, paths, lengths, evaporation_rate)
return best_path, best_length
def select_next_city(current_city, unvisited_cities, pheromone, alpha, beta):
probabilities = []
total_sum = 0
for city in unvisited_cities:
pheromone_sum = np.sum(pheromone[current_city, list(unvisited_cities)])
heuristic_value = 1 / cities[current_city][city]
probability = (pheromone[current_city, city] ** alpha) * (heuristic_value ** beta)
probabilities.append(probability)
total_sum += probability
probabilities = [p / total_sum for p in probabilities]
next_city = np.random.choice(list(unvisited_cities), p=probabilities)
return next_city
def update_pheromone(pheromone, paths, lengths, evaporation_rate):
pheromone *= (1 - evaporation_rate) # 信息素挥发
for i in range(len(paths)):
path = paths[i]
length = lengths[i]
for j in range(len(path) - 1):
city1 = path[j]
city2 = path[j + 1]
pheromone[city1][city2] += 1 / length
```
在代码中,cities是城市间距离矩阵,num_ants是蚂蚁数量,num_iterations是迭代次数,evaporation_rate是信息素挥发率,alpha和beta是启发式因子。通过调用`ant_colony_optimization`函数,可以得到最优路径(best_path)和路径长度(best_length)。
希望对你有所帮助!如有其他问题,请继续提问。
阅读全文
相关推荐
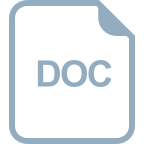
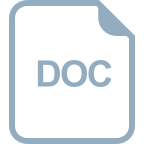
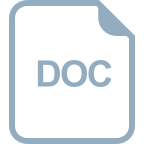

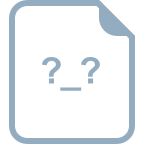
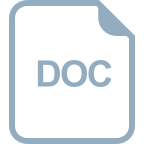

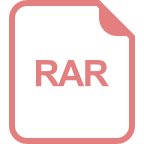
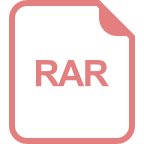
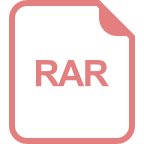
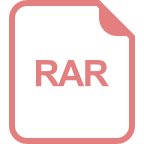
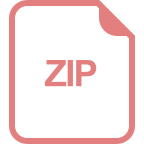
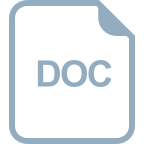





