C#读取整个文件夹的所有图片到一个数组中
时间: 2024-04-14 12:29:57 浏览: 40
您可以使用以下代码来读取整个文件夹中的所有图片,并将它们存储在一个数组中:
```csharp
using System.IO;
// 获取文件夹中的所有图片文件
string folderPath = "YourFolderPath";
string[] imageFiles = Directory.GetFiles(folderPath, "*.jpg"); // 这里假设您只想读取.jpg格式的图片文件
// 创建一个存储图片路径的数组
string[] imagePaths = new string[imageFiles.Length];
// 将图片文件的路径存储到数组中
for (int i = 0; i < imageFiles.Length; i++)
{
imagePaths[i] = imageFiles[i];
}
// 打印所有图片文件的路径
foreach (string imagePath in imagePaths)
{
Console.WriteLine(imagePath);
}
```
请将 "YourFolderPath" 替换为您要读取的文件夹路径,并确保该文件夹中包含所需的图片文件。此代码将仅读取扩展名为 .jpg 的图片文件,如果您想读取其他格式的图片文件,请相应地更改 "*.jpg" 部分的代码。
相关问题
C# 读取多个文件夹中图片并显示到图片框中
以下是一个示例代码,可以读取多个文件夹中的图片并显示在一个 PictureBox 控件中:
```csharp
private void ShowImages(string[] folderPaths)
{
List<Image> images = new List<Image>();
foreach (string folderPath in folderPaths)
{
// 获取文件夹中所有图片文件的路径
string[] imagePaths = Directory.GetFiles(folderPath, "*.jpg");
foreach (string imagePath in imagePaths)
{
// 加载图片并添加到列表中
Image image = Image.FromFile(imagePath);
images.Add(image);
}
}
// 将图片列表设置为 PictureBox 控件的 Image 属性
pictureBox1.Image = CombineImages(images);
}
private Image CombineImages(List<Image> images)
{
int width = 0;
int height = 0;
// 计算最终图片的尺寸
foreach (Image image in images)
{
width += image.Width;
height = Math.Max(height, image.Height);
}
// 创建新的 Bitmap 对象
Bitmap result = new Bitmap(width, height);
using (Graphics g = Graphics.FromImage(result))
{
int x = 0;
// 将所有图片绘制到新的 Bitmap 对象中
foreach (Image image in images)
{
g.DrawImage(image, x, 0);
x += image.Width;
}
}
return result;
}
```
调用 `ShowImages` 方法时,传入需要读取的文件夹路径的数组即可。其中 `CombineImages` 方法用于将多个图片合成为一张图片,在这里使用了 `Graphics` 类的 `DrawImage` 方法来绘制每张图片。
C# 读取图片文件夹后把图片直接显示到下拉框中
可以通过以下步骤来实现:
1. 读取图片文件夹中的所有图片文件,可以使用 `Directory.GetFiles` 方法,将文件路径存储到一个字符串数组中。
2. 创建一个 `ImageList` 控件,将读取到的每个图片文件加载到 `ImageList` 中,可以使用 `Image.FromFile` 方法。
3. 创建一个 `ComboBox` 控件,将 `ImageList` 中的图片绑定到 `ComboBox` 的 `DropDownList` 属性上,可以使用 `ComboBox.DrawMode` 属性设置为 `DrawMode.OwnerDrawFixed`,然后在 `ComboBox.DrawItem` 事件中绘制每个图片项。
以下是示例代码:
```csharp
private void Form1_Load(object sender, EventArgs e)
{
string[] imageFiles = Directory.GetFiles(@"C:\Images");
ImageList imageList = new ImageList();
foreach (string file in imageFiles)
{
imageList.Images.Add(Image.FromFile(file));
}
comboBox1.DrawMode = DrawMode.OwnerDrawFixed;
comboBox1.DropDownStyle = ComboBoxStyle.DropDownList;
comboBox1.DataSource = imageFiles;
comboBox1.ItemHeight = imageList.ImageSize.Height + 2;
comboBox1.DropDownWidth = imageList.ImageSize.Width + SystemInformation.VerticalScrollBarWidth;
comboBox1.DrawItem += ComboBox1_DrawItem;
}
private void ComboBox1_DrawItem(object sender, DrawItemEventArgs e)
{
if (e.Index < 0)
{
return;
}
ComboBox comboBox = (ComboBox)sender;
string file = (string)comboBox.Items[e.Index];
ImageList imageList = comboBox.DataSource as ImageList;
if (imageList != null)
{
Image image = imageList.Images[e.Index];
e.Graphics.DrawImage(image, e.Bounds.Left + 1, e.Bounds.Top + 1, imageList.ImageSize.Width, imageList.ImageSize.Height);
}
else
{
e.Graphics.DrawString(file, comboBox.Font, Brushes.Black, e.Bounds.Left + 1, e.Bounds.Top + 1);
}
}
```
相关推荐
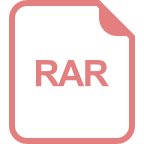













