使用C语言,并使用rsa算法求加密过程代码
时间: 2024-09-25 22:14:05 浏览: 36

C语言代码实现RSA非对称加密、解密
在C语言中实现RSA加密算法需要一些数学库的支持,比如`openssl`库。下面是一个简单的RSA加密过程的示例代码,这个例子展示了如何生成密钥对、加载公钥进行加密以及基本的字符数组加密:
```c
#include <stdio.h>
#include <string.h>
#include <openssl/rsa.h>
// 加载RSA公钥
RSA* load_public_key(const char* filename) {
RSA* rsa = NULL;
FILE* file = fopen(filename, "rb");
if (file) {
rsa = PEM_read_RSA_PUBKEY(file, NULL, NULL, NULL);
fclose(file);
}
return rsa;
}
// RSA加密函数
unsigned char* rsa_encrypt(RSA* rsa, unsigned char* data, int len) {
BIO* bio_out = BIO_new(BIO_s_mem());
unsigned char* encrypted_data;
int encrypted_len;
RSA_public_encrypt(len, data, &encrypted_data, rsa, RSA_PKCS1_OAEP_PADDING);
encrypted_len = strlen((char*)encrypted_data);
// 将加密后的数据追加到内存生物缓冲区
BIO_write(bio_out, encrypted_data, encrypted_len);
// 获取加密后的字符串并释放原始数据
encrypted_data = malloc(encrypted_len + 1);
BIO_get_mem_ptr(bio_out, &encrypted_data[0]);
encrypted_data[encrypted_len] = '\0';
// 关闭bio_out
BIO_free_all(bio_out);
return encrypted_data;
}
int main() {
RSA* rsa_public_key = load_public_key("public.pem"); // 假设你有一个名为"public.pem"的公钥文件
if (!rsa_public_key) {
printf("Failed to load public key.\n");
return -1;
}
// 示例数据,这里假设是要加密的字符串
char plain_text[] = "Hello, World!";
int plain_text_len = strlen(plain_text);
// 对字符串进行加密
unsigned char* encrypted_text = rsa_encrypt(rsa_public_key, (unsigned char*)plain_text, plain_text_len);
printf("Encrypted message: %s\n", encrypted_text); // 输出加密后的结果
// 清理
RSA_free(rsa_public_key);
free(encrypted_text);
return 0;
}
```
注意:这个示例仅用于演示目的,实际应用中你需要处理文件读取错误和其他潜在问题。并且,真正的RSA加密会涉及到更大的数值,因此通常不会直接将整个字符串作为输入。
阅读全文
相关推荐
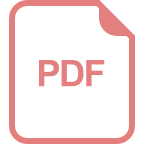
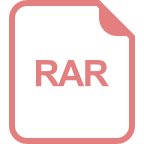
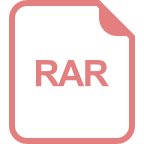
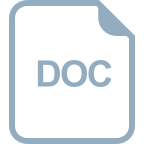












