python 字符串替换数字
时间: 2023-10-20 20:33:15 浏览: 187
在 Python 中,你可以使用 `str.replace(old, new)` 方法来替换字符串中的数字。例如,假设我们有一个字符串 `s`,想要将其中的数字替换为特定的字符,可以使用以下代码:
```python
s = "hello123world456"
new_s = ''.join(c if not c.isdigit() else '#' for c in s)
print(new_s)
```
输出:
```
hello###world###
```
在上面的示例中,我们使用了一个列表推导式来遍历字符串 `s` 中的每个字符。如果字符 `c` 是数字,则将其替换为 `'#'`,否则保留原字符。最后,我们使用 `str.join()` 方法将所有字符重新连接成一个新的字符串 `new_s`。
相关问题
python字符串替换
在Python中,字符串是不可变的,即创建后不能被修改,但是可以通过替换的方式得到一个新的字符串。Python中有多种方式进行字符串替换,以下是其中的几种:
1. replace()方法:replace()方法可以将一个指定的字符串替换为另一个字符串,语法如下:
```python
str.replace(old, new[, count])
```
其中,old表示要被替换的字符串,new表示替换后的新字符串,count表示替换的次数(可选,默认全部替换)。例如:
```python
str = "hello, world!"
new_str = str.replace("world", "python")
print(new_str) # 输出 hello, python!
```
2. re模块:re模块提供了正则表达式的支持,可以使用正则表达式进行复杂的字符串替换。例如:
```python
import re
str = "hello, world! 1234"
new_str = re.sub(r"\d+", "5678", str) # 将数字替换为5678
print(new_str) # 输出 hello, world! 5678
```
3. translate()方法:translate()方法可以将一个字符串中的每个字符替换为另一个字符,或者删除某些字符,使用时需要先创建一个转换表(translation table),然后调用translate()方法进行替换。例如:
```python
str = "hello, world!"
table = str.maketrans("el", "xy") # 创建转换表,将e替换为x,将l替换为y
new_str = str.translate(table)
print(new_str) # 输出 hxyyo, wyrd!
```
以上是Python中几种常见的字符串替换方法,可以根据实际需要选择合适的方法。
python字符串替换脚本
### Python 字符串替换示例
#### 使用 `re.sub` 函数进行正则表达式替换
通过使用Python标准库中的`re.sub`函数可以实现基于正则表达式的字符串替换操作。此功能允许指定模式来匹配目标文本并将其更换为新的内容。
```python
#!/usr/bin/env python
# encoding: utf-8
import re
url = 'https://113.215.20.136:9011/113.215.6.77/c3'
new_url = re.sub(r'(\d+\.\d+\.\d+\.)\d+', r'\1254', url)
print(new_url) # 输出更新后的URL地址
```
上述代码展示了如何利用正则表达式将IP地址的最后一部分数字更改为特定值[^1]。
#### 处理不可解码字符
当遇到编码问题时,可以通过设置错误处理机制如`\xHH`格式化方式来解决未知字节的问题:
```python
invalid_bytes = b'\xff\xfe\xfd'
decoded_string = invalid_bytes.decode('utf-8', errors='backslashreplace')
print(decoded_string) # 展现经过特殊处理后的字符串形式
```
这段程序说明了怎样安全地转换含有非法UTF-8序列的数据流[^2]。
#### 利用字符串切片技术完成局部替换
对于只需要修改字符串某一部分的情况,可以采用索引定位加切片的方式来达成目的:
```python
msg = "This is a test message containing Hello at position unknown."
position_of_hello = msg.rfind("Hello")
if position_of_hello != -1:
modified_msg = msg[:position_of_hello] + "[REPLACED]" + msg[position_of_hello+len("Hello"):]
else:
modified_msg = msg
print(modified_msg) # 显示最终被更改过的消息体
```
这里提供了一个例子展示如果找到关键词“Hello”的位置,并仅对其之后的内容做变换而不影响前面的部分[^3]。
阅读全文
相关推荐









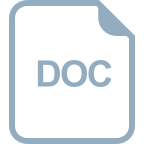



