how to calculate the triangles in multigraph with python
时间: 2024-09-20 11:02:28 浏览: 30
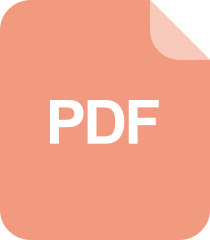
How to calculate the public psychological pressure in the social networks
In Python, calculating triangles in a multigraph (a graph that can have multiple edges between any two vertices) is a bit more complex than in a simple graph, since you need to account for all possible combinations of three nodes that are connected. Here's an outline using NetworkX library, which provides a convenient way to handle graphs:
```python
import networkx as nx
# Assuming you have a MultiGraph 'G'
G = nx.MultiGraph() # Replace this line with your actual multigraph data
def count_triangles(graph):
triangle_count = 0
for node1, neighbors1 in graph.adjacency_iter(): # Iterate over nodes and their neighbors
for node2 in neighbors1: # For each neighbor of node1
common_neighbors = set(graph.neighbors(node1)) & set(graph.neighbors(node2)) # Find common neighbors
if len(common_neighbors) >= 2: # If at least two nodes share a neighbor
triangle_count += len(common_neighbors) # Count the shared neighbors as potential triangles
return triangle_count // 6 # Since each shared edge contributes to 6 triangles, divide by 6 for uniqueness
triangle_count = count_triangles(G)
print(f"The number of triangles in the multigraph is {triangle_count}.")
```
This code iterates over all pairs of neighboring nodes and checks if they share at least two common neighbors. It counts these shared neighbors and then divides the total by 6 because each unique triangle contributes three shared edges.
Note that this method has a time complexity of O(V^3), where V is the number of vertices in the graph, so it may not be efficient for very large graphs.
阅读全文
相关推荐
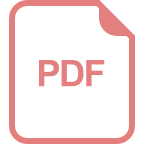
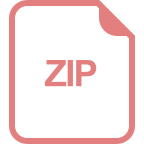















