How to calculate the highest order of a number x in C
时间: 2024-10-20 11:16:13 浏览: 5
In C programming, to find the highest order (i.e., the power of 10) that divides a given integer `x` without leaving any remainder, you can use the logarithm function or an iterative approach with division and modulo operations. Here's an example using both methods:
**Method 1: Using log10() function**
```c
#include <math.h>
int highest_order(int x) {
if (x <= 0) {
return 0; // Return 0 for negative numbers and zero
}
int exponent = floor(log10(x)); // Floor function to get whole number part
return exponent;
}
```
**Method 2: Iterative approach with division and modulo**
```c
#include <stdio.h>
int highest_order(int x) {
if (x <= 0) {
return 0; // Return 0 for negative numbers and zero
}
int order = 0;
while (x % 10 == 0) {
x /= 10; // Divide by 10 each time
order++;
}
return order;
}
```
Both functions will return the highest power of 10 that divides `x`.
阅读全文
相关推荐
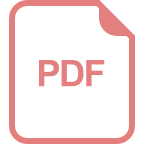
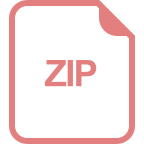
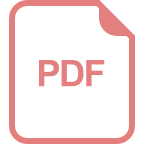















