netty TCP处理器
时间: 2023-11-02 09:07:42 浏览: 122
Netty是一个基于Java NIO的客户端/服务器框架,可以快速开发可维护的高性能协议服务器和客户端。Netty提供了一种新的方式来处理TCP连接,称为ChannelHandler,它可以轻松地处理入站和出站数据以及事件。
Netty TCP处理器的主要作用是处理TCP连接的读写事件,包括数据的编解码、粘包和拆包、心跳检测等。它可以通过ChannelPipeline来组织多个ChannelHandler,形成一个处理链,每个ChannelHandler负责处理特定的任务。
Netty TCP处理器的优点包括:
1. 高性能:基于Java NIO实现,可以支持高并发、高吞吐量的网络应用。
2. 灵活性:可以通过ChannelPipeline自由组合多个ChannelHandler,实现不同的业务逻辑。
3. 可扩展性:可以通过自定义ChannelHandler来扩展Netty的功能。
相关问题
netty 的tcp处理器
Netty 的 TCP 处理器是一个基于事件驱动的框架,它提供了高性能、可扩展性和可靠性的网络编程解决方案。它的核心是 ChannelPipeline,它是一个处理事件的链式结构,每个节点都是一个 ChannelHandler,用于处理不同类型的事件。
当一个 TCP 连接建立时,Netty 会创建一个 Channel,并将其注册到 EventLoop 上。然后,Netty 会将一系列 ChannelHandler 添加到 ChannelPipeline 中,这些 ChannelHandler 会处理不同类型的事件,例如连接建立、数据读取、数据写入等。当事件发生时,Netty 会将事件传递给 ChannelPipeline 中的第一个 ChannelHandler,然后由它决定是否将事件传递给下一个 ChannelHandler。
Netty 的 TCP 处理器还提供了许多高级特性,例如心跳检测、拆包和粘包处理、SSL 加密等。这些特性可以帮助开发者更轻松地构建高性能、可靠的网络应用程序。
netty tcp
Netty 是一款高性能、异步事件驱动的网络应用框架,可以用于快速开发可扩展的服务器和客户端程序。在 Netty 中,TCP 协议是默认支持的,因为它提供了可靠的、面向连接的数据传输方式。如果你想使用 Netty 来编写 TCP 服务器或客户端,可以通过以下步骤来实现:
1. 引入 Netty 的依赖:
```xml
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.48.Final</version>
</dependency>
```
2. 编写服务器端代码:
```java
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new YourServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture future = bootstrap.bind(port).sync();
future.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
```
3. 编写客户端代码:
```java
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new YourClientHandler());
}
});
ChannelFuture future = bootstrap.connect(host, port).sync();
future.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
```
其中,`YourServerHandler` 和 `YourClientHandler` 是自定义的处理器,用于接收和处理数据。在处理器中可以使用 Netty 提供的各种工具类来简化开发,例如 `ByteBuf`、`ChannelHandlerContext` 等。
阅读全文
相关推荐
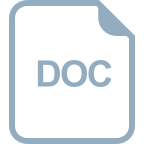
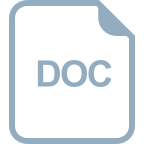
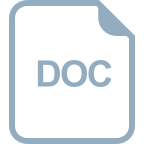
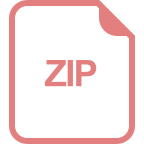
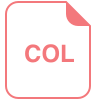









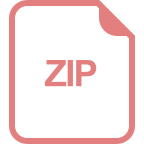
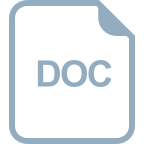
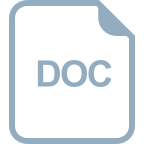