netty进行tcp通信代码
时间: 2023-09-17 12:06:00 浏览: 87
### 回答1:
这是一个基本的 netty 程序,用于建立 TCP 通信连接。
```
public class MyServer {
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new MyServerInitializer());
ChannelFuture f = b.bind(8899).sync();
f.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
```
这里是客户端代码:
```
public class MyClient {
public static void main(String[] args) throws Exception {
EventLoopGroup eventLoopGroup = new NioEventLoopGroup();
try {
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(eventLoopGroup).channel(NioSocketChannel.class)
.handler(new MyClientInitializer());
Channel channel = bootstrap.connect("localhost", 8899).sync().channel();
channel.closeFuture().sync();
} finally {
eventLoopGroup.shutdownGracefully();
}
}
}
```
这里的 `MyServerInitializer` 和 `MyClientInitializer` 是自定义的类,用于处理网络事件,例如,当客户端连接到服务器时,会触发 `channelActive` 事件。
```
public class MyServerInitializer extends ChannelInitializer<SocketChannel> {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new MyServerHandler());
}
}
```
```
public class MyClientInitializer extends ChannelInitializer<SocketChannel> {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new MyClientHandler());
}
}
```
最后,你需要实现自己的事件处理器,例如:
```
public class MyServerHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
System.out.println(ctx.channel().remoteAddress() + ": " + msg);
ctx.channel().writeAndFlush("from server: " + UUID.
### 回答2:
Netty是一个高性能、异步事件驱动的网络应用框架,用于开发可扩展的、基于TCP协议的网络通信程序。下面是使用Netty进行TCP通信的示例代码:
1. 引入Netty依赖:在项目的pom.xml文件中添加以下依赖关系:
```
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.66.Final</version>
</dependency>
```
2. 创建服务器端代码:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class Server {
public static void main(String[] args) throws Exception {
// 创建两个EventLoopGroup实例,用于处理客户端连接和处理客户端请求
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
// 创建ServerBootstrap实例,用于配置服务器相关参数
ServerBootstrap serverBootstrap = new ServerBootstrap();
serverBootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class) // 使用NIO方式处理Channel
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new ServerHandler()); // 添加自定义的ChannelHandler
}
})
.option(ChannelOption.SO_BACKLOG, 128) // 设置TCP相关参数
.childOption(ChannelOption.SO_KEEPALIVE, true);
// 绑定并监听端口
ChannelFuture channelFuture = serverBootstrap.bind(8888).sync();
// 等待服务器端口关闭
channelFuture.channel().closeFuture().sync();
} finally {
// 释放资源
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
```
3. 创建服务器端自定义处理器:
```java
import io.netty.buffer.ByteBuf;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.ChannelInboundHandlerAdapter;
public class ServerHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
ByteBuf byteBuf = (ByteBuf) msg;
try {
// 读取客户端发送的数据并进行相应处理
byte[] bytes = new byte[byteBuf.readableBytes()];
byteBuf.readBytes(bytes);
String request = new String(bytes);
System.out.println("接收到客户端请求:" + request);
// 向客户端发送响应
String response = "Hello, Client!";
byte[] responseBytes = response.getBytes();
ByteBuf responseBuf = ctx.alloc().buffer(responseBytes.length);
responseBuf.writeBytes(responseBytes);
ctx.writeAndFlush(responseBuf);
} finally {
// 释放资源
byteBuf.release();
}
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
// 异常处理
cause.printStackTrace();
ctx.close();
}
}
```
以上是一个简单的使用Netty进行TCP通信的示例代码。服务器端监听8888端口,接收客户端发送的消息,并向客户端发送响应。
### 回答3:
Netty是一个基于Java NIO的框架,用于快速开发网络通信应用程序。它提供了一种简单而强大的方式来处理各种类型的网络通信,包括TCP通信。
下面是一个使用Netty进行TCP通信的示例代码:
1. 首先,我们需要创建一个引导类来配置和启动Netty服务器:
```java
EventLoopGroup group = new NioEventLoopGroup();
try {
ServerBootstrap serverBootstrap = new ServerBootstrap();
serverBootstrap.group(group)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel socketChannel) throws Exception {
ChannelPipeline pipeline = socketChannel.pipeline();
pipeline.addLast(new StringDecoder(), new StringEncoder());
pipeline.addLast(new MyServerHandler());
}
});
ChannelFuture future = serverBootstrap.bind(8080).sync();
future.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
```
2. 接下来,我们需要实现一个自定义的服务器处理器,继承`SimpleChannelInboundHandler`类,并重写`channelRead0`方法:
```java
public class MyServerHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
// 在这里处理接收到的消息
System.out.println("Received message: " + msg);
// 响应消息给客户端
ctx.writeAndFlush("Hello from server");
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
// 处理异常
cause.printStackTrace();
ctx.close();
}
}
```
3. 最后,我们可以创建一个客户端来与服务器建立连接并发送消息:
```java
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel socketChannel) throws Exception {
ChannelPipeline pipeline = socketChannel.pipeline();
pipeline.addLast(new StringDecoder(), new StringEncoder());
pipeline.addLast(new MyClientHandler());
}
});
ChannelFuture future = bootstrap.connect("localhost", 8080).sync();
future.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
```
```java
public class MyClientHandler extends SimpleChannelInboundHandler<String> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
// 在这里处理接收到的响应消息
System.out.println("Received response: " + msg);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
// 处理异常
cause.printStackTrace();
ctx.close();
}
}
```
以上就是使用Netty进行TCP通信的简单代码示例。
相关推荐
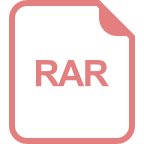
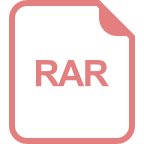














