c语言求顺序表LA和顺序表LB的交集的代码
时间: 2024-12-10 21:50:59 浏览: 8
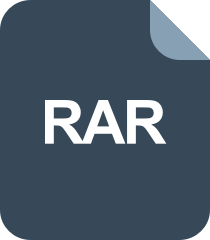
2_链表_求la和lb的交集_

在C语言中,我们可以创建一个函数来求两个顺序表LA和LB的交集。这里假设我们有一个结构体作为元素类型,并且列表已经排序。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* create_node(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 比较并添加到交集中
void find_intersection(Node* LA, Node* LB, Node** result) {
Node* intersection = NULL;
Node* la = LA, *lb = LB;
while (la && lb) {
if (la->data < lb->data)
la = la->next;
else if (la->data > lb->data)
lb = lb->next;
else { // 数据相等,添加到结果列表中
if (!intersection) *result = la; // 初始化交集头结点
intersection->next = create_node(la->data);
la = la->next;
}
}
*result = intersection; // 返回最终的交集指针
}
// 打印链表
void print_list(Node* node) {
while (node) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
// 假设LA 和 LB 已经初始化好,这里是示例数据
Node* LA = ...; // 链表LA的头结点
Node* LB = ...; // 链表LB的头结点
Node* intersection = NULL;
find_intersection(LA, LB, &intersection);
if (intersection)
printf("Intersection: ");
else
printf("No common elements.\n");
print_list(intersection); // 输出交集部分
return 0;
}
```
阅读全文
相关推荐
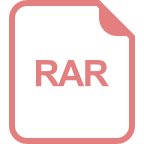
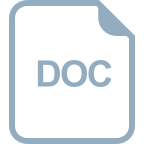
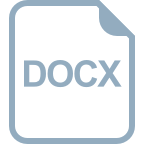
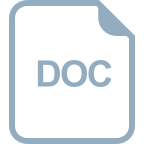
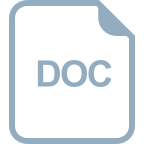
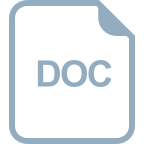
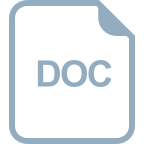





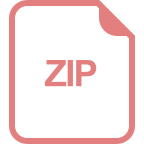
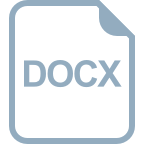