.定义一个Rectangle类,私有成员变量有height和width。请编写代码包括: (1)私有成员变量的getter()方法和setter()方法 (2)无参构造方法和有参构造方法 (3)计算面积和周长的方法 (4)输出信息的方法(分别用静态方法和实例方法实现)
时间: 2024-10-20 13:05:38 浏览: 34
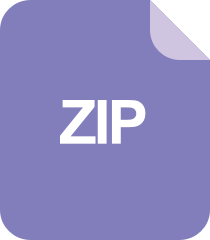
java代码-编写一个类,类名为Rectangle(矩形),它有两个整型的变量width(宽)和height(高);有一个方法area(),没有参数,返回类型为double,功能是求矩形的面积;还有另一个方法为perimeter()没有参数,返回类型为double,功能是求矩形的周长,
下面是`Rectangle`类的完整代码,包括了所请求的所有部分:
```java
public class Rectangle {
// 私有成员变量
private double height;
private double width;
// (1)私有成员变量的getter和setter方法
public double getHeight() {
return height;
}
public void setHeight(double height) {
if (height > 0) {
this.height = height;
} else {
System.out.println("Height must be a positive number.");
}
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
if (width > 0) {
this.width = width;
} else {
System.out.println("Width must be a positive number.");
}
}
// (2)无参构造方法和有参构造方法
public Rectangle() {
this(0, 0);
}
public Rectangle(double height, double width) {
if (height > 0 && width > 0) {
this.height = height;
this.width = width;
} else {
throw new IllegalArgumentException("Both dimensions must be positive numbers.");
}
}
// (3)计算面积和周长的方法
public double getArea() {
return height * width;
}
public double getPerimeter() {
return 2 * (height + width);
}
// (4)输出信息的方法(静态方法和实例方法实现)
// 静态方法
public static String printInfo(Rectangle rectangle) {
return "Rectangle information:\nHeight: " + rectangle.getHeight() + "\nWidth: " + rectangle.getWidth() +
"\nArea: " + rectangle.getArea() + "\nPerimeter: " + rectangle.getPerimeter();
}
// 实例方法
public void displayInfo() {
System.out.println(printInfo(this));
}
}
```
现在你可以创建`Rectangle`对象并调用相应的方法来操作它们:
```java
Rectangle rectangle = new Rectangle(5, 8);
rectangle.setHeight(10);
rectangle.setWidth(12);
System.out.println(rectangle.displayInfo());
```
这将会输出矩形的信息,包括面积和周长。
阅读全文
相关推荐
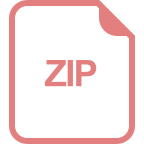
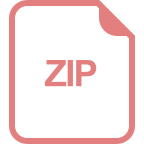





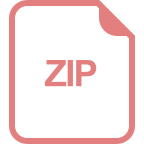

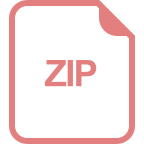
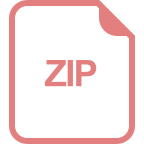
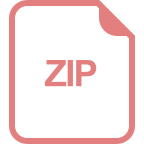
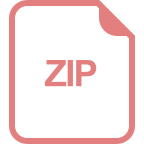
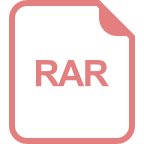