学生信息管理系统c++分大学生中学生小学生代码
时间: 2024-08-01 13:00:37 浏览: 56
在C++中创建一个学生信息管理系统(Student Information Management System, SIS),针对大学生、中学生和小学生,你需要设计一个通用的数据结构来存储学生的个人信息,并根据学龄段提供特定的操作功能。以下是基本的设计步骤:
1. 定义学生基类(Student):
```cpp
class Student {
public:
virtual ~Student() {} // 虚析构函数,确保派生类能被正确析构
string name;
int age;
virtual void displayInfo() = 0; // 纯虚函数,用于打印基本信息
};
```
2. 分别为大学生(CollegeStudent)、中学生(MiddleStudent)和小学生(PrimaryStudent)定义派生类:
```cpp
class CollegeStudent : public Student {
private:
double gpa;
public:
CollegeStudent(string n, int a) : Student(n, a), gpa(0) {}
void displayInfo() override {
cout << "姓名:" << name << ", 年龄:" << age << ", GPA:" << gpa << endl;
}
};
class MiddleStudent : public Student {
private:
vector<string> subjects;
public:
MiddleStudent(string n, int a) : Student(n, a) {}
void displayInfo() override {
cout << "姓名:" << name << ", 年龄:" << age << ", 学科:" << join(subjects, ", ") << endl;
}
};
class PrimaryStudent : public Student {
private:
list<char> grades;
public:
PrimaryStudent(string n, int a) : Student(n, a) {}
void displayInfo() override {
cout << "姓名:" << name << ", 年龄:" << age << ", 成绩:" << join(grades, ", ") << endl;
}
};
```
3. 在主程序中管理这些对象:
```cpp
int main() {
Student* college = new CollegeStudent("张三", 20);
college->displayInfo();
Student* middle = new MiddleStudent("李四", 15);
middle->displayInfo();
Student* primary = new PrimaryStudent("王五", 8);
primary->displayInfo();
delete college;
delete middle;
delete primary;
return 0;
}
```
阅读全文
相关推荐
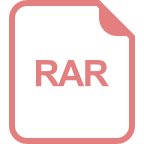
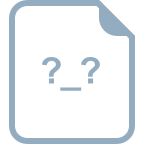
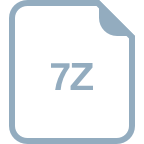


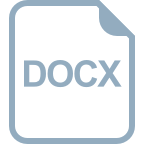
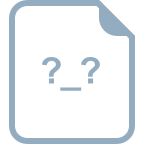
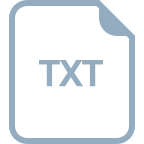
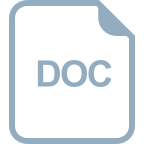
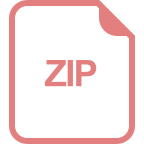
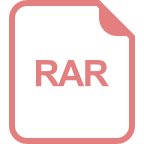
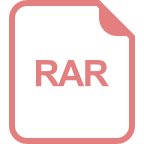
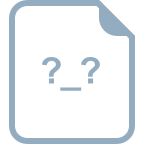
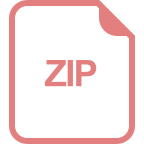
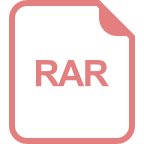
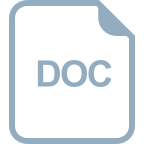
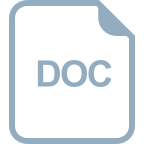
