unity如何控制transform中的rotation使得角色反转
时间: 2024-12-19 17:29:06 浏览: 45
在Unity中,如果你想要控制`Transform`中的`rotation`,使其让角色反转,你可以通过设置`Rotation`属性来改变其朝向。假设你有一个`GameObject`,名为`myCharacter`,它的`Transform`包含一个`rotation`字段,你可以这样做:
```csharp
// 获取当前旋转
Quaternion currentRotation = myCharacter.transform.rotation;
// 创建一个新的旋转,通常用90度的正交旋转表示反转
Quaternion reverseRotation = Quaternion.Euler(0, 180, 0); // 对于沿Y轴反转
// 将新的旋转应用到角色
myCharacter.transform.rotation = reverseRotation;
// 或者如果你想在一段时间内平滑地反转,可以使用`Transition Euler`或`Animate Quaternion`
myCharacter.transform.Rotate(0, 180, 0, Time.deltaTime);
相关问题
unity animation动画帧反转
在Unity中,可以通过以下步骤实现动画帧的反转:
1. 首先,在Inspector视图中选中相关的Animation动画。
2. 找到Root Transform Rotation,并更改它的Offset为适当的角度。如果动画在X轴方向相反,则可以将Offset设置为180度。
3. 点击Apply按钮应用更改即可。
这样设置之后,动画帧就会被反转,实现了动画的镜像效果。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [[Unity][Animation&Animator]人物动画方向相反,调整旋转动画方向](https://blog.csdn.net/BuladeMian/article/details/78985099)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *3* [Unity3D逐帧播放动画脚本1](https://download.csdn.net/download/weixin_35748716/86362294)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
MPU6050输出俯仰角,反转角,航向角控制UNITY小球运动
要实现MPU6050输出俯仰角,反转角和航向角控制UNITY小球运动,需要进行以下步骤:
1. 连接MPU6050传感器和Arduino板。
2. 使用Arduino IDE编写代码,读取MPU6050的数据并计算俯仰角,反转角和航向角。
3. 将计算得到的角度数据通过串口传输到Unity中。
4. 在Unity中创建小球模型,并编写脚本,接收通过串口传输的角度数据,控制小球的运动。
以下是示例代码:
Arduino代码:
```
#include <Wire.h>
#include <MPU6050.h>
MPU6050 mpu;
void setup() {
Serial.begin(9600);
Wire.begin();
mpu.initialize();
}
void loop() {
float ax, ay, az;
float gx, gy, gz;
mpu.getMotion6(&ax, &ay, &az, &gx, &gy, &gz);
float roll = atan2(ay, az) * 180 / PI;
float pitch = atan2(-ax, sqrt(ay * ay + az * az)) * 180 / PI;
float yaw = atan2((gz * sin(pitch) - gy * cos(pitch)), (gx * cos(roll) + gy * sin(roll) * sin(pitch) + gz * sin(roll) * cos(pitch))) * 180 / PI;
Serial.print("Roll: ");
Serial.print(roll);
Serial.print(" Pitch: ");
Serial.print(pitch);
Serial.print(" Yaw: ");
Serial.println(yaw);
delay(100);
}
```
Unity代码:
```
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO.Ports;
public class MPU6050Control : MonoBehaviour {
SerialPort sp = new SerialPort("COM3", 9600);
void Start() {
sp.Open();
sp.ReadTimeout = 1;
}
void Update() {
if (sp.IsOpen) {
try {
string data = sp.ReadLine();
string[] angles = data.Split(' ');
float roll = float.Parse(angles[1]);
float pitch = float.Parse(angles[3]);
float yaw = float.Parse(angles[5]);
Vector3 rotation = new Vector3(-pitch, yaw, roll);
transform.rotation = Quaternion.Euler(rotation);
} catch (System.Exception) {
}
}
}
void OnApplicationQuit() {
sp.Close();
}
}
```
在Unity中创建一个空对象,将上述代码添加到该对象上,然后将小球模型作为其子对象,并将脚本拖到空对象上。
通过上述步骤,就可以实现MPU6050输出俯仰角,反转角和航向角控制UNITY小球运动。
阅读全文
相关推荐
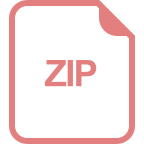
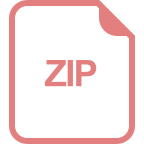
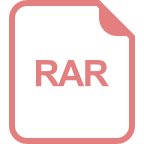
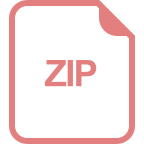
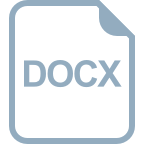
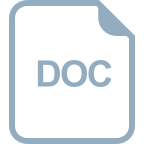
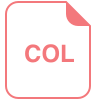
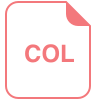





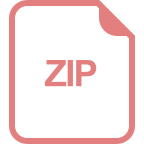
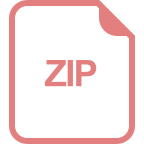