unity物体沿着Y轴在一定范围内来回旋转15度
时间: 2023-10-13 10:04:24 浏览: 50
可以通过以下代码实现:
```csharp
using UnityEngine;
public class RotateObject : MonoBehaviour
{
public float rotationRange = 15f;
public float rotationSpeed = 1f;
private float rotationAngle;
void Update()
{
rotationAngle += rotationSpeed * Time.deltaTime;
if(rotationAngle > rotationRange || rotationAngle < -rotationRange)
{
rotationSpeed *= -1f;
}
transform.rotation = Quaternion.Euler(0f, rotationAngle, 0f);
}
}
```
这个脚本会让物体绕着 Y 轴进行旋转,旋转的范围是 `rotationRange` 度,旋转的速度是 `rotationSpeed` 度每秒。当物体旋转到了范围的边界时,旋转的方向会反转。我们通过 `Quaternion.Euler` 方法来创建旋转的四元数,其中 Y 轴的旋转角度为 `rotationAngle`。
相关问题
unity物体沿着Y轴在一定范围内来回旋转5度
你可以使用以下代码来实现这个功能:
```csharp
public class RotateObject : MonoBehaviour
{
public float rotationRange = 10f;
public float rotationSpeed = 5f;
private bool rotateClockwise = true;
void Update()
{
// 根据旋转方向计算旋转角度
float rotationAmount = rotateClockwise ? rotationSpeed : -rotationSpeed;
// 沿着Y轴旋转物体
transform.Rotate(new Vector3(0f, rotationAmount, 0f) * Time.deltaTime);
// 如果物体达到旋转范围的边界,改变旋转方向
if (transform.rotation.eulerAngles.y >= rotationRange && rotateClockwise)
rotateClockwise = false;
else if (transform.rotation.eulerAngles.y <= 0 && !rotateClockwise)
rotateClockwise = true;
}
}
```
在这个脚本中,我们定义了旋转的范围(`rotationRange`)和旋转速度(`rotationSpeed`),以及一个布尔值变量 `rotateClockwise`,用于记录当前物体的旋转方向。在 `Update()` 函数中,我们使用 `transform.Rotate()` 函数来沿着 Y 轴旋转物体,并根据当前的旋转方向计算旋转角度。如果物体达到旋转范围的边界,我们就改变旋转方向,使其沿着相反的方向旋转。
unity物体沿着某个轴在一定范围内来回旋转15度
可以通过以下代码实现:
```csharp
using UnityEngine;
public class RotateObject : MonoBehaviour
{
public float rotationRange = 15f;
public float rotationSpeed = 1f;
public Vector3 rotationAxis = Vector3.up;
private float rotationAngle;
void Update()
{
rotationAngle += rotationSpeed * Time.deltaTime;
if(rotationAngle > rotationRange || rotationAngle < -rotationRange)
{
rotationSpeed *= -1f;
}
transform.rotation = Quaternion.AngleAxis(rotationAngle, rotationAxis);
}
}
```
这个脚本会让物体绕着 `rotationAxis` 轴进行旋转,旋转的范围是 `rotationRange` 度,旋转的速度是 `rotationSpeed` 度每秒。当物体旋转到了范围的边界时,旋转的方向会反转。
相关推荐
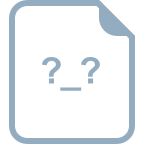












