data.iloc提取第六列除第一行外的全部数据
时间: 2024-04-15 09:29:29 浏览: 120
你可以使用`data.iloc`方法来提取第六列除第一行外的全部数据。以下是示例代码:
```python
import pandas as pd
# 假设你的数据存储在一个名为data的DataFrame中
# 提取第六列除第一行外的全部数据
extracted_data = data.iloc[1:, 5]
# 如果你想将提取的数据存储在一个新的DataFrame中
new_data = pd.DataFrame(extracted_data, columns=['Column 6'])
```
这样,`extracted_data`将包含第六列除第一行外的全部数据。如果你想将提取的数据存储在一个新的DataFrame中,可以使用`pd.DataFrame`方法创建一个新的DataFrame,并指定列名。
相关问题
帮我检查以下代码的问题file_path = '粤雷渔08888.csv' data = pd.read_csv(file_path,encoding='gbk',header=0) # 将日期列转换为datetime类型 data['日期'] = pd.to_datetime(data['日期']) # 计算相邻日期的差值 diff = data['日期'].diff() # 将间隔为1日的日期分为一组 group = (diff != pd.Timedelta(days=1)).cumsum() # 分组并提取数据 dfs = [group for _, group in data.groupby(group)] # 输出结果 for i, df in enumerate(dfs): df['日期'] = df['日期'].map(change_date1) for i, df in enumerate(dfs): index = 0 while index < df.shape[0]: if (df.iloc[index, 5].split(":")[0] == "23" and int(df.iloc[index, 5].split(":")[1]) >= 30 and df.iloc[index+1,4].split(":")[0] == "00" and int(df.iloc[index+1,4].split(":")[1]) <= 12): df.loc[index] = [df.iloc[index,0],df.iloc[index,1],df.iloc[index,2]+df.iloc[index+1,2],df.iloc[index,3]+"--"+df.iloc[index+1,3],df.iloc[index,4],df.iloc[index+1,5],df.iloc[index,6].split("-")[0]+"-次日"+df.iloc[index+1,6].split("-")[-1]] index += 1 else: df.drop(index = index,inplace=True) if index == df.shape[0]: break print(f"Dataframe {i+1}:") print(df) if not os.path.exists('粤雷渔088881.csv'): df.to_csv('粤雷渔088881.csv', encoding='gbk', mode='a', index=False, index_label=False) else: df.to_csv('粤雷渔088881.csv', encoding='gbk', mode='a', index=False, index_label=False, header=False)
这段代码的问题在于文件路径中包含了中文字符,可能会导致读取文件失败。建议将文件名改为英文或者使用相对路径。另外,读取文件时指定了编码为GBK,需要确保文件的编码也是GBK。header=0表示第一行为列名。
# K近邻算法 from sklearn import neighbors # 导包 from sklearn.model_selection import train_test_split import pandas as pd data = pd.read_csv("data/预处理.csv.", header=None); X = data.iloc[:, 1:14] # 0到124行;1-14列,训练集 Y = data.iloc[:, 0] Xtrain, Xtest, Ytrain, Ytest = train_test_split(X, Y, test_size=0.3) # 测试集占30% clf = neighbors.KNeighborsClassifier(n_neighbors=3, weights='distance') # 实例化对象 训练模型 clf.fit(Xtrain, Ytrain) # 拟合数据 # predict = clf.predict(Ytrain) print("准确率为:", clf.score(Xtest, Ytest))
这段代码使用了scikit-learn库中的K近邻算法来进行分类任务。下面是对代码的解释:
1. 首先,导入了需要的库:`neighbors`模块用于K近邻分类器,`train_test_split`函数用于数据集的划分,以及`pandas`库用于数据处理。
2. 接下来,使用`pd.read_csv`函数从CSV文件中读取数据,并将其存储在名为`data`的DataFrame对象中。
3. 通过`data.iloc`方法,从`data`中提取特征变量`X`(列1-14)和目标变量`Y`(第0列)。
4. 使用`train_test_split`函数将数据集划分为训练集和测试集,其中测试集占总数据的30%。划分后的数据分别存储在`Xtrain`、`Xtest`、`Ytrain`和`Ytest`中。
5. 创建一个K近邻分类器对象,并使用`n_neighbors=3`设置最近邻居的数量,`weights='distance'`设置权重计算方式为距离加权。
6. 使用`clf.fit`方法拟合训练数据,训练模型。
7. 通过调用`clf.score`方法计算在测试集上的准确率,并使用`print`语句打印结果。
注意:代码中的`predict`变量被注释掉了,因此并没有使用。如果你需要获取预测结果,可以将其取消注释,并将数据集改为`Xtrain`。
阅读全文
相关推荐
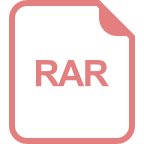
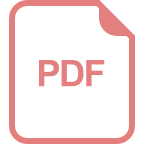
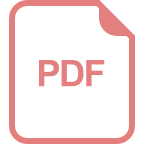













