同步fifo verilog
时间: 2025-01-08 09:21:52 浏览: 2
### 同步FIFO设计与实现
#### 1. 设计概述
同步FIFO(First In First Out)是一种常见的硬件结构,广泛应用于数据流控制中。其主要目的是解决不同模块间的数据传输速率不匹配问题[^2]。
#### 2. Verilog代码示例
下面是一个简单的同步FIFO的Verilog实现:
```verilog
module sync_fifo #(
parameter DATA_WIDTH = 8,
parameter FIFO_DEPTH = 16
)(
input wire clk, // Clock signal
input wire rst_n, // Asynchronous reset (active low)
input wire wr_en, // Write enable
input wire rd_en, // Read enable
input wire [DATA_WIDTH-1:0] din, // Data to write into the FIFO
output reg [DATA_WIDTH-1:0] dout, // Data read from the FIFO
output wire empty, // FIFO is empty flag
output wire almost_empty,// Almost empty flag
output wire full // FIFO is full flag
);
// Internal signals and registers declaration
reg [$clog2(FIFO_DEPTH):0] w_ptr; // Write pointer with extra bit for wrap-around detection
reg [$clog2(FIFO_DEPTH)-1:0] r_ptr_reg; // Registered read pointer
wire [$clog2(FIFO_DEPTH)-1:0] r_ptr_next;
wire [$clog2(FIFO_DEPTH):0] r_ptr_ext;
assign r_ptr_next = rd_en ? r_ptr_reg + 1'b1 : r_ptr_reg;
assign r_ptr_ext = {r_ptr_next[$clog2(FIFO_DEPTH)-1], r_ptr_next};
// Memory array instantiation
reg [DATA_WIDTH-1:0] mem[FIFO_DEPTH-1:0];
// Write operation
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
w_ptr <= 'd0;
end else if (wr_en && !full) begin
mem[w_ptr[$clog2(FIFO_DEPTH)-1:0]] <= din;
w_ptr <= w_ptr + 1'b1;
end
end
// Read operation
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
r_ptr_reg <= 'd0;
dout <= 'b0;
end else if (rd_en && !empty) begin
dout <= mem[r_ptr_reg];
r_ptr_reg <= r_ptr_next;
end
end
// Status flags generation
assign empty = (w_ptr == r_ptr_ext);
assign almost_empty = ((w_ptr - r_ptr_ext) < 2);
assign full = (w_ptr[$clog2(FIFO_DEPTH)] != r_ptr_ext[$clog2(FIFO_DEPTH)]) &&
(w_ptr[$clog2(FIFO_DEPTH)-1:0] == r_ptr_ext[$clog2(FIFO_DEPTH)-1:0]);
endmodule
```
此段代码定义了一个参数化的同步FIFO模块,其中`DATA_WIDTH`指定数据宽度,`FIFO_DEPTH`设定队列深度。该模块包含了基本的操作接口如读写使能、输入输出端口以及状态标志位等。
#### 3. 测试平台(Testbench)
为了验证上述同步FIFO的功能正确性,可以构建如下测试平台来进行仿真:
```verilog
module tb_sync_fifo();
parameter CLK_PERIOD = 10; // ns
reg clk;
initial forever #(CLK_PERIOD/2) clk = ~clk;
reg rst_n;
reg wr_en;
reg rd_en;
reg [7:0] din;
wire [7:0] dout;
wire empty;
wire almost_empty;
wire full;
sync_fifo #(.DATA_WIDTH(8), .FIFO_DEPTH(16)) uut (
.clk(clk),
.rst_n(rst_n),
.wr_en(wr_en),
.rd_en(rd_en),
.din(din),
.dout(dout),
.empty(empty),
.almost_empty(almost_empty),
.full(full)
);
initial begin
$dumpfile("tb_sync_fifo.vcd");
$dumpvars(0, tb_sync_fifo);
// Initialize all inputs
clk = 0;
rst_n = 0;
wr_en = 0;
rd_en = 0;
din = 0;
// Apply reset pulse
repeat(5) @(negedge clk);
rst_n = 1;
// Perform some operations on the FIFO...
// For example, perform a series of writes followed by reads.
// Add your test cases here.
// Finish simulation after tests are done
$finish();
end
// Example stimulus process can be added below this line...
endmodule
```
这段Testbench脚本设置了时钟周期,并创建了必要的信号来驱动DUT(Design Under Test)。它还提供了重置脉冲以确保初始条件一致。用户可以根据具体需求添加更多刺激过程来全面检验FIFO的行为特性[^4]。
阅读全文
相关推荐







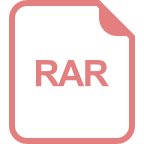
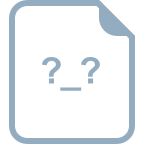





